IEnumerable is an interface which is implemented by collections.It is used to iterate over the collection elements.
Some of the features of this interface are:
- It is used to iterate over a collection in forward direction.
- It can not be used to modify collection elements.
- A collection implementing this interface can use foreach loop to iterate the collection elements.
- It is used to execute LINQ queries on in memory collections.
- So IEnumberable should be implemented by a collection class when the collection needs to be iterated using foreach loop.Most of the collection classes such as list implements this interface.
IQueryable is an interface which inherits IEnumerable interface.There are some differences between IEnumerable and IQueryable interfaces:
- While IEneumberable is implemented by collections which wants to execute queries in memory,IQueryable is implemented by collections which wants to execute remote queries such as on database server.
- While IEneumberable is used for LINQ to Objects queries,IQueryable is used for LINQ to SQL queries.
- In IQueryable, LINQ queries are executed on the remote server such as database server unlike IEnumberable which executes queries in-memory

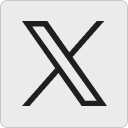