Name:Chain of responsibility design pattern
Type:Behavioral Design Pattern
The Chain of responsibility design pattern is a behavioral pattern.It is useful when you want to give more than one handler chance to handle the request.
The request is passed along a chain of handlers until it is handled by one of the handlers in the chain or none of handlers is able to handle the request.The response is then returned to the client.
Another feature of the Chain of responsibility pattern is that the request is decoupled from the handler.
Example of Chain of responsibility design pattern
Following is an example of Chain of responsibility pattern.We are taking example of e-commerce application which has multiple supply agents for different products.User can request different types of items.These items can be supplied by any of the supply chain agents.
As you can observe we are passing the request for item to a chain of different handlers.Handlers here are supply chain agents.If a request for an item is not handled by one handler ,which is supply agent ,then it is passed to the next handler.
Item class represents an item which is sold by the e commerce company.
public class Item { public string Type { get; set; } }
We are defining the abstract class which represents different request handlers as:
abstract class RequestHandler { protected RequestHandler successor; public void SetSuccessor(RequestHandler successor) { this.successor = successor; } public abstract void HandleRequest(Item mobile); } class ElectronicsSupplyAgent : RequestHandler { public override void HandleRequest(Item item) { if (item.Type=="electronics") { Console.WriteLine("requesnt handled by ElectronicsSupplyAgent"); } else if (successor != null) { successor.HandleRequest(item); } } }
We are defining supply chain agents which sell different items as derived classes of Request Handler:
class BooksSupplyAgent : RequestHandler { public override void HandleRequest(Item item) { if (item.Type == "books") { Console.WriteLine("request handled by BooksSupplyAgent"); } else if (successor != null) { successor.HandleRequest(item); } } } class ClothesSupplyAgent : RequestHandler { public override void HandleRequest(Item item) { if (item.Type == "books") { Console.WriteLine("request handled by BooksSupplyAgent"); } else if (successor != null) { successor.HandleRequest(item); } } }
The above classes define the main components of the Chain of responsibility design pattern.
We call the handlers in our main method as:
RequestHandler electronicsSupplyAgent = new ElectronicsSupplyAgent(); RequestHandler booksSupplyAgent = new BooksSupplyAgent(); ClothesSupplyAgent clothesSupplyAgent = new ClothesSupplyAgent(); List<RequestHandler> handlers = new List<RequestHandler>(); electronicsSupplyAgent.SetSuccessor(booksSupplyAgent); booksSupplyAgent.SetSuccessor(clothesSupplyAgent); handlers.Add(electronicsSupplyAgent); handlers.Add(booksSupplyAgent); handlers.Add(clothesSupplyAgent); Item item = new Item { Type = "electronics" }; handlers.ForEach(o => o.HandleRequest(item));
It is a very basic example of Chain of responsibility design pattern.You can create different implementations of this pattern depending upon the scenario.

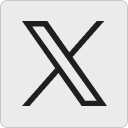