using System;
using System.Collections.Generic;
namespace CSharpAlgos
{
class Program
{
static void Main(string[] args)
{
string name="abczcba";
bool pal=true;
//if the string contains even characters
if(name.Length%2==0)
{
//each character should occur even number of times
Dictionary<char,int> dic=newDictionary<char, int>();
foreach(var ch inname)
{
if(!dic.ContainsKey(ch))
dic.Add(ch,0);
dic[ch]=dic[ch]+1;}
foreach(KeyValuePair<char,int> kv indic)
{
if(kv.Value%2!=0)
pal=false;
Console.Write(kv.Value);
}}
else
{
int count=0;
//each character should occur even number of times
Dictionary<char,int> dic=newDictionary<char, int>();
foreach(var ch inname)
{
if(!dic.ContainsKey(ch))
dic.Add(ch,0);
dic[ch]=dic[ch]+1;}
foreach(KeyValuePair<char,int> kv indic)
{
if(kv.Value%2!=0)
{
count++;
if(count==2)
pal=false;
}
Console.Write(kv.Value);
}
}
Console.WriteLine(pal);
}
}
}

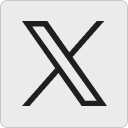
Leave a Reply