As.NET developers, often we work with data from various sources. Suppose you are working on some HR application where you have some employee IDs within your database list and employee information coming through some API. Now you want to find out whether data from this API contains some of the employees in your database or not. In such a scenario we need to combine data from multiple sources.
Matching Elements in Two Lists in C#
To implement this, we can loop through the list of employees and check if any employee’s `Id` is matching with any of the IDs in the list of Ids We can convert the string list into a set for fast lookups: If found – return the corresponding Employee object; otherwise return null.
public class Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Id { get; set; }
}
//Search Employee by Id in a list
public class FindEmployee
{
public static Employee? FindMatchingEmployee(List<string> listOfEmployeeIds, List<Employee> listOfEmployees)
{
// Convert string list to a HashSet for faster lookups
HashSet<int> employeeIdsSet = listOfEmployeeIds.Select(id => int.Parse(id)).ToHashSet();
// Iterate through the employee list
foreach (var employee in listOfEmployees)
{
if (employeeIdsSet.Contains(employee.Id))
{
return employee; // Return the matching employee
}
}
return null; // Return null if not found
}
}
Following is the above implementation step by step:
1. Define the Employee Class: The Employee class has three properties: FirstName,LastName, and Id. These represent the details of each employee.
2. Convert the String List to a HashSet: The list of employee IDs (as strings) is converted to a HashSet<int> after parsing each string to an integer. The HashSet provides faster lookup times for comparison.
3. Iterate Through the Employees: Loop through each Employee object in the listOfEmployees to check if its Id is present in the HashSet of IDs.
4. Return the Result:
If a match is found, return the Employee object.
If no match is found after the loop, return null
5. Usage of the Method: Call this method by passing `listOfEmployeeIds` and `listOfEmployees` to find a matching employee or confirm that no matches exist.
This approach ensures readability and efficiency, making it suitable for scenarios where performance and clarity are both essential.

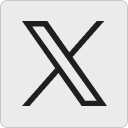
Leave a Reply