Toast notification is a mechanism in Android application to display a message for short duration to the user.The Toast notification is displayed on top of the current screen and occupies partial screen space.It is like a popup in windows applications.It goes away itself after a short duration.
To display toast notification in Android you need to call the following two methods of the Toast object:
- makeText()
- show()
To display the toast notification first create and set properties of the toast object using the following code:
Toast.makeText(context, message, length).show();
- context : Represents an activity
- message: Message that is to be displayed to the user
After this show the toast notification using the show() method:
toast.show();
Custom Toast Notification
Define the view to be used with toast:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/custom_toast" android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:id="@+id/text" android:layout_width="wrap_content" android:layout_height="fill_parent" android:textColor="#000000" /> </LinearLayout>
Use the LayoutInflater,which connects the view with the viewgroup, using the following code:
LayoutInflater inflater = getLayoutInflater(); View layout = inflater.inflate(R.layout.custom_toast, (ViewGroup) findViewById(R.id.container));
After this we are setting the text of the text layout by using the following code:
TextView text = (TextView) layout.findViewById(R.id.text); text.setText("This is a custom toast");
Finally we are creating a toast object:
Toast toast = new Toast(getApplicationContext()); toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0); toast.setDuration(Toast.LENGTH_LONG); toast.setView(layout); toast.show();

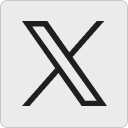
Leave a Reply