SQLite is a RDBMS(relational database management system).There are many other RDBMS available such as Oracle, MySQL and SQL Server.SQLite differs from these RDBMS since it is not a client server database management system.Unlike other RDBMS SQLite is a embedded RDBMS.
SQLite is embedded as a part of the the mobile application.This means that you don’t need to install or setup SQLite to use it.You manage RDBMS by directly executing commands.This allows it to be a very lightweight RDBMS and suitable for embedded applications.
Here we will understand how to perform read and update operations using SQLite.
We will use a Java class SQLiteOpenHelper for performing different operations on the SQLite database.We will create a subclass derived from this class.The sublcass will implement the methods onCreate(SQLiteDatabase) and onUpgrade(SQLiteDatabase, int, int)
onCreate method is called when database is created.
onUpgrade You call this method when the database scheme needs to be modified.
Create a sub class deriving from the SQLiteOpenHelper class
public class DatabaseHandler extends SQLiteOpenHelper {…
import the following packages
android.database.sqlite.SQLiteOpenHelper
android.database.sqlite.SQLiteDatabase
In the constructor call the super class constructor.Also pass the database name and version as arguments
public DatabaseHandler(Context context) { super(context, "SampleDatabase", null, 1); }
Override the onCreate method.Create the tables required in this method.
@Override public void onCreate(SQLiteDatabase db) { String EMP_TABLE ="CREATE TABLE Employees ("ID INTEGER PRIMARY KEY,NAME TEXT,DEPARTMENT TEXT)";db.execSQL(EMP_TABLE); }
Override the onUpgrade method if required
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { // db.execSQL(ALTER TABLE COMMAND); // onCreate(db); }

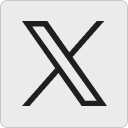
Leave a Reply