Angular 2 is a client side JavaScript platform for developing web and mobile applications.It is a complete rewrite of angular 1.So Knowing angular 1 is not required to learn angular 2.
Following are some of the important points about Angular 2:
- It is used for creating dynamic html application.Angular 2 applications are a tree of Components.
- Uses ES6 and typescript
- Angular differs completely from Angular 1.Things such as scope and controller ,which are a part of angular 1 , are not included in angular 2.
- uses reactjs
- Uses Typescript for writing applications.TypeScript has many additional features which are not available in javascript.TypeScript can be used for object oriented programming,static type checking.
Since its our first Angular 2 application so we will have look at just some of the basic concepts which we will require to build our application.
Following are the main concepts in Angular 2:
- Module Module is a package of components,directives and services.
- Component It contains logic and UI.It is the basic building block of angular 2 application.
- Bootstrapping It is the process of starting or launching Angular application.
- Decorators These are used to add metadata.For example to make a class as component we use the @Component() decorator.
- Import statements Import statements are used to import classes defined in modules.For example to import component from @angular2/core we use: import {component} from ‘@angular2/core’;
Angular 2 application has a hierarchy of folders since Angular 2 application is a tree of components.All the components are defined in app root folder:
app
component1
component1.html
component1.ts
component2
component2.html
component2.ts
All the components are defined inside separate folders in app folder.
We will create a very simple angular 2 application to understand the basics.
Since we will be calling our application from a browser,so we need an html page.This page will launch our application.We will just look at the main parts of the different files.The complete source code of the files is declared at the end.
We will create index.html page.We will include the following script in our page.
<script> System.import('main.js').catch(function(err){ console.error(err); }); </script>
This script is calling the main.js file.This file is compiled to javascript from the typescript file main.ts.
Main.ts file is the entry point to angular2 application ,since it contains the bootstrap logic.
It contains the following line which starts the module called AppModule.
platformBrowserDynamic().bootstrapModule(AppModule);
Modules in TypeScript are just containers for components.AppModule contains the following declaration in the NgModule decorator.
bootstrap: [ AppComponent ]
The above line tells angular 2 to start the AppComponent and that it is the root component of our application.
Finally the AppComponent is the class which defines the following selector
selector: ‘my-app’
If you remember this is what we have declared in the index.html page.This selector informs angular to instantiate this component when my-app element is declared in the html page.
index.html Browser displays this html page.This page in turn calls the angular 2 application by using the selector defined by the angular component.
<html> <head> <title>Angular Sample Application</title> <script src="systemjs.config.js"></script> <script> System.import('main.js').catch(function(err){ console.error(err); }); </script> <body> <my-app></my-app> </body> </html>
This is what we call from index.html:
main.ts
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic'; import { AppModule } from './app/app.module'; platformBrowserDynamic().bootstrapModule(AppModule);
app.module.ts This is the bootstrap module called from the above file.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; @NgModule({ imports:[ BrowserModule ], declarations: [ AppComponent ], bootstrap: [ AppComponent ] }) export class AppModule { }
app.component.ts AppComponent contains the application logic.Here it contains the logic to display some text.
import { Component } from '@angular/core'; //this is used to import Component class from @angular/core module. @Component({ selector: 'my-app', template: `<h1>Hello from {{name}}</h1>` }) export class AppComponent { name = 'Angular'; }
On executing the above application following text is displayed on the screen:
Hello from Angular

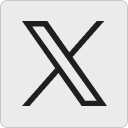
Leave a Reply