To make HTTP Requests in Angular we use the methods of HttpClient class which is defined in the @angular/common/http package. HttpClient uses XMLHttpRequest browser API behind the scenes.
HttpClient class provides the following methods each corresponding to the different HTTP verbs:
- get
- post
- put
- delete
- patch
- head
- jsonp
HttpClient provides several methods for making HTTP requests to the server.One of the methods is POST which is used for making POST requests to the server.All methods of the HttpClient class returns an object of Observable type.To execute the HTTP request subscribe to this Observable instance.
Before using the HttpClient class in your application define it as a dependency in the constructor of the component.
constructor(private http: HttpClient) { }
HttpClient.POST method accepts URL, request body and optional http options as arguments.For example to make POST request to the URL http://www.employees.com
call the POST method as:
http.post<Employee>('http://www.employees.com', { employeeId:1, Name:'FirstEmp' } );
Now define the above logic in a method called AddEmployee:
addEmpoyee(employee: Employee): Observable<Employee> { return http.post<Employee>('http://www.employees.com', { employeeId:1, Name:'FirstEmp' } ); }
finally to make the HTTP request to the server subscribe to the above method:
this.addEmpoyee(employee).subscribe( employee=> { console.log(employee); } );

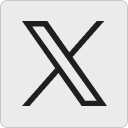
Leave a Reply