HTML is designed to display static documents.So if a web site contains just static documents then HTML is sufficient for such a site.But web applications of today are much more than just a group of static documents.Web applications are expected to perform lot of processing on the client.To provide user interactivity web applications use client side frameworks such as jQuery.These client side frameworks manipulate the HTML DOM to implement the required behaviour on the client.
In contrast to the popular frameworks that manipulate the DOM of HTML ,AngularJS provides the client interactivity by extending HTML rather than manipulating it.Directives are an important part of AngularJS which allows us to attach custom behaviours to the DOM elements such elements,attributes and css.
Directives allows us to remove duplication of behaviour.We can create a directive for reusable behaviour and use that directive instead of copying the markup at many different places.
While working with AngularJS applications we use lot of built in directives.Some of the commonly used built in directives in AngularJS are:
- ng-app Defines AngularJS application
- ng-controller Attaches controller to a DOM element
- ng-bind Specifies expression to use for the content of HTML element
- ng-click Specifies behavior for HTML element click event
- ng-repeat Used to create HTML template for every item in a collection of items
Creating custom directives
We can create these types of custom directives.Each directive determines how the directive will be activated
- Element Directive is activated if there is a matching HTML element with the directives name
- Attributes Directive is activated if there is a matching attribute
- CSS Directive is activated if there is a matching CSS class
- Comment Directive is activated if there is a matching comment
Element directives are most commonly used.Though other directives are useful depending upon the scenario.
In the following example we will create an element directive
<script> myapp = angular.module("app", []); myapp.directive('helloDirective', function() { var directive = {}; directive.restrict = 'E'; /* restrict this directive to elements */ directive.template = "My first directive"; return directive; }); </script>
In the first line we create a new angular module called app.This module calls the directive() function .This directive() function takes two arguments:
- First is the name of the directive.Here we have named our directive as helloDirective
- Second parameter is a factory function which returns the directive object.The directive object is a javascript object with some properties.Inside the factory function we set the properties of this directive object.Two of the important properties we need to set for this directive object are restrict and template.
The ‘restrict’ property determines how the directive is matched to HTML.The valid values for restrict property are
- E directive will be activated if there is matching HTML element
- A directive will be activated if there is matching HTML attributes
- EA directive will be activated if there is matching HTML element or HTML attribute
The ‘template’ property determines the content of the matched HTML element.So if a HTML element matched the directive then the contents of the template property replaces the contents of the element.
Though we have assigned a static text value to the ‘template’ property but it is more useful to assign expressions to the template.We can assign expression to the template property as:
directive.template = "Welcome {{user}}";
When the above directive is rendered the expression {{user}} is replaced with the value of user as assigned in the scope.
To activate the above directive we can use the following
<body> <div ng-app="app" > <hello-Directive></hello-Directive> </div> </body>
Another useful property is templateUrl.This specifies the path to the template.We can define template to use in a HTML file and assign the path to the templateUrl property.For example if our template is defined in a file called customtemplate.html then we can assign it to the templateUrl property as:
templateUrl: ‘customtemplate.html’
Naming convention of AngularJS directive
We name the directive using the camel case syntax.In camel case syntax every word except the first begins with a capital letter.AngularJS converts element or attribute name to match the directive name ,a process known as normalization.
In the normalization process “-” ,”:” or “_” delimited names are converted to camelCase
So if we name our directive as helloWorld and it is an Element directive then it will be activated using the following elements
<hello-world></hello-world> <hello:world></hello:world> <hello_world></hello_world>
In the normalization process angularjs automatically converts the “-” or other separator character to the CamelCase directive name.This is the reason that we find the names of the AngularJS directives using the CamelCase while when we use them we use the hyphen “-“.For example ngBind is the name of AngularJS directive used for rendering expression as the text value of an HTML element.But when we use this directive we use it as ng-bind=”expression”.

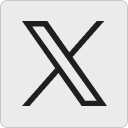
Leave a Reply