The ngOptions attribute can be used to dynamically generate a list of <option> elements for the <select> element
So if you have an array of items then you can bind it to select element ng-options directive.To bind ng-options to array of values we just need to add the ng-options directive to a select element.We can use the ng-options directive as:
<select ng-model="Model" ng-options="item.property for item in Array">
as it is clear from the above syntax Array is the array we want to bind the select item to ,item represents the elements in the Array while item.property is the property in the item which we want to bind to the select element.In the case of simple type we will just bind to the element and not specify item.property.
For example we can bind to simple string values as:
$scope.selectedEmp= "1" $scope.employees = ["1","2","3","4","5"];
and in the model we specify:
<div ng-app="myApp" ng-controller="myCtrl"> {{selectedEmp}} <select ng-model="selectedEmp" ng-options="emp for emp in employees"> </select> <label ng-model="selectedEmp"></label> </div>
you can observe that when you select an item in the combobox then displayed value next to it is automatically updated.This is because we are using the expression {{selectedEmp}} to display the value selected in the combo box.
For more complex types you can specify the property in the ng-options.In the following example we are binding to employees objects where each employee has id and name.
we have declared an array of employees as:
$scope.employees = [ {name:'emp1', id:'1'}, {name:'emp2', id:'2'}, {name:'emp3', id:'3'}, {name:'emp4', id:'4'}, {name:'emp5', id:'5'} ];
You can bind the above employees array to select as:
<select ng-model="selectedEmp" ng-options="emp.name for emp in employees">
the above syntax means that every item in employees array will be iterated and bound to the select element.Every item is represented as emp and we are binding the emp.Name property of every employee.
If we want to bind the id property of every empployee then we can just use emp.id instead of emp.name
<select ng-model="selectedEmp" ng-options="emp.id for emp in employees">
Following is the remaing code
<div ng-app="myApp" ng-controller="myCtrl"> <select ng-model="selectedEmp" ng-options="emp.id for emp in employees"> </select> <label ng-model="selectedEmp"></label> </div> <script> var app = angular.module('myApp', []); app.controller('myCtrl', function($scope) { $scope.employees = [ {name:'emp1', id:'1'}, {name:'emp2', id:'2'}, {name:'emp3', id:'3'}, {name:'emp4', id:'4'}, {name:'emp5', id:'5'} ]; }); $scope.selectedEmp=$scope.employees[1]; </script>
For setting the default value in ng-options just specify the ng-model value to the item which you want to select by default.

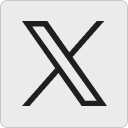
Leave a Reply