Middle ware is a software component which gets request and generates response. Middleware always receives request ,but it can do the following with the request:
- pass the request to the next component in the pipeline
- generate and return response
ASP.NET Core MVC application is created as a Console application.This is because MVC Core application is created using middleware.We can combine multiple middlewares to form a sequence.There are two important classes in any ASP.NET Core application:
- Program class for configuring the application infrastructure
- Startup class for configuring the middleware.It defines the ConfigureServices and Configure methods:
public void ConfigureServices(IServiceCollection services) { } public void Configure(IApplicationBuilder app) { }
Program class is like a normal program class of a console application.It consists of a Main() method.
To create MVC pipileine we use the Use__ extension methods in the configure method of Startup class.You use an object of IApplicationBuilder type to add these middleware.
These methods adds a middleware to the pipeline.For example to use the Authentication middleware you use the UseAuthentication extension method in the Configure method of the Stratup class as:
public void Configure(IApplicationBuilder app) { app.UseAuthentication(); }
If you want to add any other middleware to the pipeline you would call the appropriate extension method.The middlewares are called in the same sequence they are added in the pipeline.
We can create a sample asp.net core application as:
Select File–>New Project
select .NET Core and ASP.NET Core 2.0 in the dropdowns.Select Web Application(MVC) in the project template list.
After the ASP.NET Core project is created you can see the Program and Startup class as:
Program class is defined as:
public class Program { public static void Main(string[] args) { BuildWebHost(args).Run(); } public static IWebHost BuildWebHost(string[] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>() .Build(); }
Main method is declared in a Program class.ASP.NET Core application execution starts from the Main method just like a console application.BuildWebHost creates and returns IWebHost instance.The IWebHost is important part of any ASP.NET Core application as it consists of Kestrel server and the configuration of the application.IWebHost is configured using WebHostBuilder.The CreateDefaultBuilder method creates an instance of WebHostBuilder.
Startup class is defined as:
public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddMvc(); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseBrowserLink(); app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); } app.UseStaticFiles(); app.UseMvc(routes => { routes.MapRoute( name: "default", template: "{controller=Home}/{action=Index}/{id?}"); }); }
Configure method is used for creating the request pipeline.The ConfigureServices method is used for adding services to the container.Here we are adding just the Mvc service.

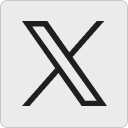
Leave a Reply