.NET MAUI framework for cross platform development
.NET Multi-platform App UI commonly known as MAUI ,is a cross platform framework that allows developers to create applications which run on multiple operating systems and uses the same codebase across the platforms. Since it supports Android, iOS, macOS, and Windows, this makes it a powerful app development framework for building applications. With MAUI, you can leverage the .NET ecosystem and C# to develop native applications.
Differences between .NET MAUI on Windows and macOS
While .NET MAUI is designed to provide a unified development experience, there are some differences between its implementations on Windows and macOS:
- Windows-Specific Features While in Windows, MAUI uses the Windows UI Library (WinUI) for rendering, on macOS, it uses Mac Catalyst to bridge iOS and macOS development.
- Development Environment While Windows users can use Visual Studio 2022 with full debugging and hot reload support, macOS users require command line and VS Code, as VS for mac has been discontinued by Microsoft.
- Device Emulation While Android emulators are available on both Windows and macOS, but for iOS development a Mac system is required.
- Native API Integration macOS apps can integrate with Apple’s APIs via Mac Catalyst, whereas Windows applications rely on Windows-specific APIs.
Even though there are these differences across the platforms, most of the MAUI application logic remains unchanged across platforms.
Installing .NET MAUI on macOS
You can use these steps to install and set up .NET MAUI on a Mac:
1. Install Homebrew (if not already installed)
Homebrew is a package manager for macOS that simplifies software installation. Open the Terminal and run:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
2. Install .NET SDK
Download and install the latest .NET SDK (at least .NET 8) from Microsoft’s official site. You can also install it via Homebrew:
brew install dotnet
3. Install Xcode and Command Line Tools
Since MAUI requires Xcode for macOS and iOS development, install it from the Mac App Store or via the command line:
xcode-select --install
After installation, agree to the license:
sudo xcodebuild -license accept
4. Install MAUI Workload
Once .NET SDK is installed, install the MAUI workload using the following command:
dotnet workload install maui
5. Verify the Installation
Run the following command to ensure everything is set up correctly:
dotnet --list-sdks
dotnet workload list
At this step you should be able to see .NET MAUI listed among the installed workloads.
6. Set Up an IDE (Visual Studio Code or JetBrains Rider)
Since Visual Studio for Mac is discontinued, you can use Visual Studio Code with the C# Dev Kit extension or JetBrains Rider as alternatives.
- Download VS Code from here.
- Install the C# extension from the marketplace.
7. Create and Run a .NET MAUI Project
Now, create a new MAUI project using the terminal:
dotnet new maui -n MyMauiApp
cd MyMauiApp
dotnet build
dotnet run
This will build and launch your .NET MAUI application on macOS.
.NET MAUI allows both windows and mac developers to build cross platform apps using C# and .NET. While there are some differences in how MAUI runs on Windows vs. macOS, the core development experience remains same.
Follow on:
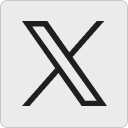