What is Dependency Injection in ASP.NET Core
ASP.NET Core provides a simple prebuilt DI container.We need to register the dependencies in this container.Dependencies could be the custom classes or the framework classes
Once you have registered the dependency in the container you can inject it anywhere you need.The DI container will automatically inject the dependencies where you have injected the dependencies For example if you registered the dependency as below,then the DI container will automatically create the object corresponding to the IEmployee and inject it into the constructor
void SimpleMethod(IEmployee employee) { }
How do you register dependencies
You add the dependency using the ConfigureServices method of the Startup class
public void ConfigureServices(IServiceCollection services) { //Add dependency to the services collection }
One common extension method that you use for working with MVC application is services.AddMvc() ,this method adds the required dependencies for working with MVC.
You can either write the extension method of a library,if it provides one or you can register the dependency in the container as:
services.AddScoped<IEmailSender, EmailSender>();
When a dependency is injected in a component ,either a new instance of the dependent object is created or an existing object is reused.This depends upon the service lifetime we define for the dependency.We can define the following lifetimes of a dependency:
You can add services as:
- Scoped new object will be created for every client request
- Singleton same object will be used every time
- Transient dependent objects are created every time they are requested and are never shared.
How do you use it
You just need to specify the dependency as a constructor parameter,and the required dependency will be created and injected by the .net core framework:
public class TestController : Controller { IEmailSender _emailSender; public HomeController(IEmailSender emailSender) { _emailSender= emailSender; } }

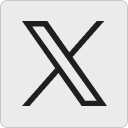