Enum type provides the GetName() and GetNames() methods which we can use to Convert Enum to String in C#.
Enum.GetName() method
GetName() method returns an equivalent string value for a given enum value.
We have declared the following enum EmployeeDepartment:
public enum EmployeeDepartment { IT, HR, Admin }
If you have a variable of type EmployeeDepartment then you can convert it to equivalent string value by using the GetName() method as:
static void Main(string[] args) { EmployeeDepartment empDept = EmployeeDepartment.IT; string name = Enum.GetName(typeof(EmployeeDepartment),empDept); }
Above program will print the following:
Enum.GetNames() method
GetName() method returns equivalent string values(string array) for a given enum.If we want to retrieve string values for the enum EmployeeDepartment declared above then we can use the following logic:
string[] empTypes = Enum.GetNames(typeof(EmployeeDepartment));

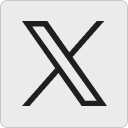