MVC is used for developing web applications just like ASP.NET WebForms.The main difference between ASP.NET MVC and ASP.NET WebForms is MVC is used for developing loosely coupled applications.
MVC applications have many advantages over WebForms applications such as
- Loose coupling of the application components
- Separation of concerns
- MVC application are easier to unit test
- MVC applications are easier to maintain and extend
Though MVC offers advantages such as the ones mentioned above,but it suffers from one draw back.Since MVC doesn’t have the concept of built in controls ,so developers are responsible for implementing all the HTML.This can be a tedious process ,especially when you are repeating the same HTML in different views.
For many built in HTML controls MVC includes built in helper methods.You can learn about these from HTML Helper methods
If we have a requirement for some custom HTML which is not provided by these inbuilt helper methods then we can create our own custom html helper methods.
To create HTML helper method we need to follow the below steps.
1.Declare a static class
2. Declare a static method in the static class.This is our extension method and the first parameter usually is HtmlHelper.
In the following example we have declared a helper method.This html helper will add a custom css class and set the title attribute which is passed in as a parameter.
namespace TestApp.CustomHelpers { public static class CustomHelpers { public static IHtmlString MyImageHelper(this HtmlHelper helper, string title) { TagBuilder tb = new TagBuilder("input"); tb.Attributes.Add("class","AppClass" ); tb.Attributes.Add("title",title ); return new MvcHtmlString(tb.ToString(TagRenderMode.SelfClosing)); } } }
This is a very simple example to help understand how to create custom html helpers in MVC and custom html helper methods can be used for more complex scenarios.

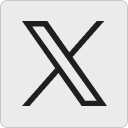