There are multiple ways to loop over list of items in TypeScript.You select the method depending upon your requirement.For example you can use the for loop to iterate over all the items in a collection.
Looping from start index to end index
For looping over elements from start limit to max limit we use for loop with a counter variable.The syntax of this loop is:-
for(var counter:number = 1; number<1; number++){ // }
For example ,to loop over elements from 1 to 100 we can use for loop as:
for(var limit:number = 1; limit<100; limit++){ alert("for loop executed : " + limit) }
Looping over collection
If you have a collection which contains different items then you can loop over the collection using for ..in loop as:-
For collections we normally use
for(var item in collection) { alert(collection[item]); }
this will loop over all the items in the collection.So if your requirement is to loop over all the collection elements then you could use this loop.
Suppose you have declared a string array as:
var coll: string[]; coll = ["a","b","c","d","e"];
then you can loop over all the items in the array:
for(var item in coll) { alert(coll[item]); }
Another way of looping over collection elements
Another option for looping over the items in the collection is item of collection ,used as:
for example if you have declared collection as:
coll = ["a","b"]; //then you can loop over the collection elements as: for (let alphabet of coll) { alert(alphabet); }
you can also loop over string using this loop:
coll = "ab"; for (let char of coll) { alert(character); }

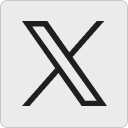