TypeScript is a language which is superset of the popular scripting language Javascript.This means that TypeScript supports all the features provided by Javascript and also adds its own set of useful features.As TypeScript is developed by Microsoft so it is well supported in Visual Studio.
Today javascript is supported across different platforms such as web browsers,web servers and even some desktop applications are built using javascript.But using Javascript to build large applications can be difficult.
TypeScript makes it easier to develop large scale Javascript applications.To achieve this it adds features missing in Javascript such as:
- Strong typing
- Classes and Modules
- Interfaces
- Generics
TypeScript supports the ECMAScript standard ,which is the standard that defines the features of Javascript.So TypeScript supports all the features of javascript.This also means that all Javascript applications are also TypeScript applications.
Ultimately TypeScript programs are compiled to javascript.The TypeScript compiler converts the TypeScript code into javascript.
Some of the important points about TypeScript are depicted
Some of the benefits provided by TypeScript are:
TypeScript helps build large applications which spans thousands of lines.Though large applications can be developed using Javascript but managing the complexity of such applications can be quite challenging.TypeScript provides features such as classes and interfaces to manage the complexity.
As Javascript is an interpreted language so it is not type checked.If there are any type errors they are either not detected or worse they are caught at run time.TypeScript being a compiled language catches any compilation errors.So if there are any compilation errors they are caught at compile time.
Following Javascript has type error which is caught only at runtime:
var count1=5; var count2=6; count1="Hello World"; var sum=count1+count2;
It supports the javascript frameworks such as jQuery,AngularJS.So we can develop TypeScript programs using these libraries.Not only can a TypeScript program use these javascript libraries ,it has the ability to strongly type the Javascript libraries.It achieves this by using a file with .d.ts extension.
These files contain the metadata of the javascript libraries.This means that if some method in the library expects an int type then it can not be called with a string type parameter.
Example TypeScript application
TypeScript programs are defined in a file having an extension .ts .When we create a new project using the TypeScript template then by default a file is created called app.ts .In the example application we have modified the contents of the file as:
class SampleApp { element: HTMLElement; constructor(element: HTMLElement) { this.element = element; this.element.innerHTML = "Hello World!"; } } window.onload = () => { var el = document.getElementById('content'); var student = new SampleApp(el); };
On running the application we get a message in the browser as:

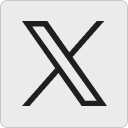