Anonymous types are a way to create objects of a type which we don’t need to access multiple times.The types we define in any application are supposed to be used multiple
times during the lifetime of the application.There are scenarios where we need to access a type only once.For this type of scenario we usually define a temporary type.
This temporary type we define is supposed to be used only once.So it’s not very useful to define a type such as a class to be used only once.
We can use anonymous types in such scenarios.Anonymous type doesn’t have a name.We define anonymous type as:
var User= new { Name = "John", TelephoneNo=12325633 };
The above line defines an anonymous type with two properties Name and TelephoneNo.The anonymous type object is assigned to the variable temp.The properties Name and TelephoneNo doesn’t have a type.The types of the properties is automatically inferred by the compiler depending on the value assigned to them.
Following are the attributes of anonymous type
- Anonymous type doesn’t have a name.The name is automatically generated by the compiler ,but we don’t have access to this name.
- The only members which can be defined by an anonymous type are public read only properties.
- The only way to access anonymous type is through an implicitly typed variable.We declare such a variable using the var keyword.Since anonymous types dosen’t have any name so we can not assign anonymous type to any other type of variable.
- Anonymous type is created using Object initialization syntax.In Object initialization syntax we assign values to the properties of an object at the time of its creation.
If we don’t use anonymous type then we will have to declare a class even if we require it for temporarily storing the information.
class User { public string Name{get;} public int TelephoneNo{get;} public string Address{get;} public int Id{get;} }
By using anonymous type we can avoid declaring such a class.We can store the same information as the above class by using the following anonymous type
var User= new { Name = "John", TelephoneNo=12325633,Address="XYZ",Id=1 };
Array of anonymous type
We can create array of anonymous type using implicitly type array as:
var Users= new[] { new { Name= "Mark", TelephoneNo=1245345 }, new { Name= "Tim",TelephoneNo=1267532} }
Anonymous types are commonly used in LINQ queries.We use anonymous type to define a type in the select part as:
var userList = from user in users select new { usr.Name, user.Id};
The user in the above query represents a single user.We can select just a few properties from the user type using the anonymous type.So the user type may have 4 properties but we are selecting only the Name and Id properties above.
Anonymous type in C# was introduced in version 3.0 and is useful when working with other language features such as Linq.

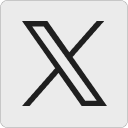

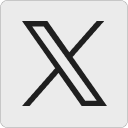

Leave a Reply