Attributes are one of the main features of c#.Attributes can be used if we want to attach metadata to the program elements such as class or method.Attributes are used with reflection to retrieve the metadata at runtime.
Attributes are classes which are derived from the System.Attribute class which is an abstract class.Though there are lot of different attributes provided by the framework for different functionalities ,we can create more attributes by deriving from the Attribute class if required.Some of the attributes provided by the framework are
Conditional This is used with methods and used to determine whether the method should execute.So if we attach the following attribute with a method then the method will execute for a debug build but not for the release build.
[Conditional("DEBUG")]
Obsolete This is used to inform the user of the program element that the program element is no longer supported and the user should rather use a different version or element.
Serializable This is used to mark a class as serializable
Caller Info Attributes These are used to obtain the information about the caller of a method. CallerMemberNameAttribute for instance is used to obtain information about the calling method.
If the inbuilt attributes does not meet our requirements then we can easily create new attributes by declaring a new class deriving from the Attribute class.
Attributes in c# simple example
To create a new attribute we need to follow the following steps
1. Declare a new class deriving from the abstract Attribute class .In the below code we have defined an Emplyee attribute which inherits from the Attribute class.
class EmployeeAttribute : Attribute { }
2. Declare a constructor having parameters we want to pass to the attribute as arguments.These constructor parameters represents the positional parameters.These are mandatory to pass when we attach the attribute to the program element.In the attribute class we have added the constructor with a single parameter which represents the positional parameter.
class EmployeeAttribute : Attribute { public string Name; public EmployeeAttribute(string name) { this.Name=name; } }
3. Declare field or properties for the parameters we want to pass to the attribute class.These represents the named parameters and are optional.These are called named parameters as we need to pass these parameters by name when attaching the attribute with the program element.
We have added the the property Address.This represents the named parameter.
class EmployeeAttribute:Attribute { public string Name; public string address; public string Address { get { return address; } set { address=value; } } public EmployeeAttribute(string name) { this.Name=name; } }
So we have created a new attribute Employee which we can apply to any program element.
If we want to restrict our attribute to certain program element then we need to add the following attribute to our attribute class
[AttributeUsage(AttributeTargets.Class)]
The above code will restrict the attribute target to a class.AttributeTargets is an enum defined in the System namespace.Some of the members in the AttributeTargets enum are
- Assembly
- Class
- Struct
- Method
- Property
Now let’s see how we can apply the attribute to a program element.
Attaching attributes to a program entity
We can apply the attribute to a program element by placing the attribute name in square [] brackets before the program element. But to apply the attribute to an assembly we need to use the below syntax
[assembly: Attribute("argument")]
This we need to add to the AssemblyInfo.cs file.
Below we have created a class AttributeTest to which we have applied the Employee attribute. To apply the attribute we just need to place the attribute in square brackets before the program element.Though we have used EmployeeAttribute name while declaring our class,we don’t need to add the suffix attribute while attaching the attribute to the program entity.
[Employee("tiger")] class AttributeTest { public void Welcome() { Console.WriteLine("Hi there!"); } }
We can retrieve the information about the program element and access the attached attributes using reflection.This Using reflection in C# gives an overview about how to use reflection.

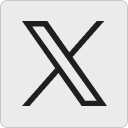
Leave a Reply