When we work with strings we sometimes need to verify the validity of input string.As the user can input any string so we need to verify if the input contains valid characters.Here we will validate the user entered input for checking string for balanced parentheses in C#
There could be different reasons to validate the input such as to verify if it contains any illegal characters or to verify if the input is as per our business rules.We will validate the user entered input to verify if the string is well formed or not by checking parentheses.
In this scenario we are validating if the input contains the proper braces or not.But we can expand on the logic and apply it to any other input as well.
In the following VerifySyntax method we are validating if the user entered values are well formed.
static bool VerifySyntax(string[] values) { int curlyBrace = 0; int roundBrace = 0; int squareBrace = 0; foreach (var item in values) { if (item == '{') curlyBrace = curlyBrace + 1; else if (item == '}') curlyBrace = curlyBrace - 1; else if (item=='[') squareBrace = squareBrace + 1; else if (item == ']') squareBrace = squareBrace - 1; else if (item == '(') roundBrace = squareBrace + 1; else if (item == ')') roundBrace = squareBrace - 1; } if (curlyBrace == 0 && squareBrace == 0 && roundBrace == 0) return true else return false }

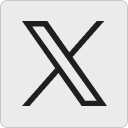
Leave a Reply