To Compare two generic lists in c# using linq ,we need to compare some of the properties of the generic lists.
So if we have two generic lists declared as:
List<string> lstA = new List<string>{ "A", "B", "C" }; List<string> lstB = new List<string> { "A", "B", "C" };
then we can compare the lists for equality by using the following logic:
1.Compare the types of generic lists
2.Compare the number of elements in the list
3.Compare if both the lists consists of common items
We can implement the above logic as:
//get the type of elements in the list var a = lstA.GetType(); var b = lstB.GetType(); //check if the types are same if (a == b) { //check if the count is same if (lstA.Count() == lstB.Count()) { //check if both the lists contains the common items var filteredSequence = lstA.Where(x => lstB.Contains(x)); if(filteredSequence.Count()==lstA.Count()) { //lists are same } else { //lists are not same } } }
We can move the above logic in a method as:
private static bool CompareGenericLists(List<string> lstA, List<string> lstB) { var a = lstA.GetType(); var b = lstB.GetType(); if (a == b) { if (lstA.Count() == lstB.Count()) { var filteredSequence = lstA.Where(x => lstB.Contains(x)); if (filteredSequence.Count() == lstA.Count()) { return true; } else { return false; } } } else { return false; } return false; }

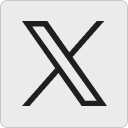
Leave a Reply