As we know method overloading means that class can define different versions of the same method.This means a method with the same name can be defined with different signatures in the same class.
For example we can define following methods called Add in a class
public Class Calculate { public int Add(int a,int b) { return a+b; } public float Add(float a,float b) { return a+b; } }
The Add method is overloaded in the Calculate class.Similarly we can overload constructors in a class.
For example a class called Employee can define the following constructors
public class Employee { public Employee() { //assign values } public Employee(string Name, string EmpId) { if(!string.IsNullOrEmpty(Name) && !string.IsNullOrEmpty(EmpId)) //assign values } public Employee(string Name, string EmpId, string Department) { if(!string.IsNullOrEmpty(Name) && !string.IsNullOrEmpty(EmpId) && !string.IsNullOrEmpty(Department)) //assign values } }
Different constructors will be invoked depending on the parameters passed when creating objects of the Employee class.
Employee emp=new Employee();//parameterless constructor Employee emp=new Employee("Marc","1");//Name,EmpId constructor Employee emp=new Employee("Marc","1","33");//Name,EmpId,Department constructor
Though the above method of invoking the individual constructors is fine there is one problem in the above code.The assignment logic in the constructor is duplicated.If there are more validation rules then there will be more code duplication in the constructors.
Constructor chaining in C#
The above class can be defined by using constructors chaining.Constructor chaining means that one constructor calls another constructor.
To call another constructor in the same class the keyword this is used.So the Employee class can be defined as:
public class Employee { public Employee():this(string.Empty,string.Empty,string.Empty) { Console.WriteLine("Employee() constructor called"); } public Employee(string Name, string EmpId):this(Name,EmpId,string.Empty) { Console.WriteLine("Employee(Name,EmpId) constructor called"); } public Employee(string Name, string EmpId, string Department) { Console.WriteLine("Employee(Name,EmpId,Department) constructor called"); if (!string.IsNullOrEmpty(Name) && !string.IsNullOrEmpty(EmpId) && !string.IsNullOrEmpty(Department)) ; //assign values } }
Now instead of duplicating the assignment and validation logic in all the constructors we move it to the single constructor.This also makes the code easier to maintain and understand.
When constructors are chained like this different constructors are called in a sequence which is
“The chained constructor is called before the invoking constructor”
So in the above example the constructor Add(string Name, string EmpId, string Department) will be called before the other two constructors.
If we create objects of the Employee class as:
Employee emp1 = new Employee(); Employee emp2 = new Employee("Marc", "32");
we will get the following message:
Employee(Name,EmpId,Department) constructor called
Employee() constructor called
Employee(Name,EmpId,Department) constructor called
Employee(Name,EmpId) constructor called

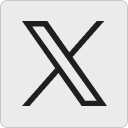
Leave a Reply