While working with strings two of the most common requirements are to convert string to title and Sentence case .We can use inbuilt and custom methods in c# for these tasks.
We will see how to convert string to Title and Sentence Case in C#.
Following are two of the methods for converting string:
Convert String to Title Case
Sometimes we have the requirement while working with textual data to convert the text into title case.If we just want to convert every word in the text to title case then we can use the ToTitleCase method.The ToTitleCase(string) is a method defined in the TextInfo class of the System.Globalization namespace.
To convert the string to title case we just pass the string to the ToTitleCase(string) method.Below we are passing some string value to this method
static void Main(string[] args) { string text = "This is a sample text"; string titleCaseText = System.Globalization.CultureInfo.CurrentCulture.TextInfo.ToTitleCase(text); Console.WriteLine(sentence titleCaseText); Console.ReadLine(); }
On executing the above code we get the following output
Convert String to Sentence Case
Though we can use the ToTitleCase(string) method for converting a string to a title case but when working with a paragraph we need to convert the first word of a sentence to title case.For this we can use the following code.
class Program { //Create a new delegate type public delegate string FormatString(string rawString); static void Main(string[] args) { string strParagraph = "first sentence.second sentence"; FormatString format = RemoveSpace; string formatted = RemoveSpace(strParagraph); FormatString split =Split; var finalString = Split(formatted); Console.WriteLine(finalString); Console.ReadLine(); } public static string RemoveSpace(string fullString) { return fullString.Trim(); } public static string Split(string fullString) { var strArr = fullString.Split(new char[] { '.' }); var newStrArr=strArr; foreach (var item in strArr) for(int iCount=0;iCount<strArr.Count();iCount++) { strArr[iCount]=strArr[iCount].Insert(0, strArr[iCount][0].ToString().ToUpper()); strArr[iCount] = strArr[iCount].Remove(1, 1); } return string.Join(".", strArr); } }
In the above example a delegate type FormatString is declared as:
public delegate string FormatString(string rawString);
The method RemoveSpace is assigned to delegate FormatString and is called.
FormatString format = RemoveSpace; string formatted = RemoveSpace(strParagraph);
the result of the first method call is passed to the second second delegate instance.The second instance refers to the Split method.
FormatString split = Split; var finalString = Split(formatted);
In the Split(string) method the passed argument is split based on the “.” separator.
var strArr = fullString.Split(new char[] { '.' });
Finally we loop over the array and convert the first word to title case.
strArr[iCount] = strArr[iCount].Insert(0, strArr[iCount][0].ToString().ToUpper());
we get the following output on executing the above example

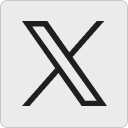
Leave a Reply