Many applications provides the registration option to the users.While providing details as a part of the registration process apart from the username and password,user is also required to provide the email address.Asking the user for the email address as a part of the registration process is good option since it can be used in the case of password recovery.
After the user provides the email address it should be verified.Verification of the email address ensures that the user has provided the correct email address.Once the user verifies the email his account is activated.
Here we will see how to implement the user email verification process.Following are the steps in the email verification process:
- User enters his registration details such as userid,email address,password
- Users details are saved in the database and his status is marked as inactive
- User is sent a confirmation email with verification link
- On clicking the link his status is updated as Active in the application
We implement the above steps as:
1.Create a table ‘Users’ in the database for storing the user details
Create Users table using the following script CREATE TABLE [dbo].[Users]( [UserName] [varchar](50) NULL, [Email] [varchar](50) NULL, [Password] [varchar](50) NULL, [Id] [varchar](max) NULL, [IsActive] [bit] NULL ) ON [PRIMARY] TEXTIMAGE_ON [PRIMARY]
2.When the user registers in the application user’s details are saved in the ‘Users’ database table.We can use stored procedure to insert the entered user details.Here we have created InsertUserDetails stored procedure as:
Create PROCEDURE InsertUserDetails ( @UserName VARCHAR(10), @Email VARCHAR(10), @Password VARCHAR(10), @UniqueId, @result int OUTPUT ) AS BEGIN insert into Users(UserName,Email,Password,Id) values( @UserName,@Email,@Password,UniqueId)
You generate the UniqueId using the following code:
string uniqueId = Guid.NewGuid().ToString();
3.User is sent an email with the verification link.When the user visits the confirmation link his status becomes as active.
Create the link by using the activation code:
SendMail(){ int SendStatus = 0; try { MailAddress fromAddress = new MailAddress(from, fromDisplayName);//red from config var toAddress = new MailAddress(MailTo, ""); string fromPassword = mailPassword;//read from config string subject = MailSubject; string body = MailBody; var message = new MailMessage(fromAddress, toAddress); message.Subject = subject; string link = HttpContext.Request.Url.GetLeftPart(UriPartial.Authority) + "/Authentication/Verify/id="+uniqueId ; message.Body = string.Format("{0} {1} {2} {3}", "Click the following link to activate your account", link, System.Environment.NewLine, "admin"); message.IsBodyHtml = true; smtp.Send(message); SendStatus = 1; return SendStatus; } catch(Exception exp) { return SendStatus; } }
Finally verify the activation link when the user clicks on it.When the user exists you update the status of the user to active in the database: We define a VerifyUserAndUpdate method which will be called when the controller corresponding to the link is clicked
public bool VerifyUserAndUpdate(string id) { bool userExists; using (SqlCommand cmd = new SqlCommand("VerifyUser")) { using (SqlDataAdapter sda = new SqlDataAdapter()) { cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.AddWithValue("@UserId",id ); SqlParameter resultParam= new SqlParameter("@result", System.Data.SqlDbType.Int); OutputParam.Direction = ParameterDirection.Output; cmd.Parameters.Add(resultParam); cmd.Connection = con; con.Open(); userId = Convert.ToInt32(cmd.ExecuteScalar()); con.Close(); } } int.TryParse(resultParam.Value.ToString(),out returnValue); if(returnValue==1) userExists=true; else userExists=false; return userExists; }
In the VerifyAndActivateUser stored procedure we check if the user exists in the database.If the user exists we change his status to active.
Create PROCEDURE VerifyAndActivateUser( @UserName VARCHAR(10), @result int OUTPUT) AS BEGIN IF EXISTS (select UserName from Users WHERE Id=@UniqueId) BEGIN UPDATE Users SET IsActive=1 WHERE Id=@UniqueId set @result=1 END else set @result=0

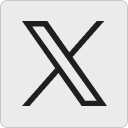
Leave a Reply