
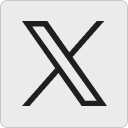

Some of the basic questions asked in interviews are:
What is an Interface in C# ?
An interface has no implementation; it only has the signature or in other words, just the definition of the methods without the body.Interface is implemented by a class.If a class implements an interface then it needs to implement all the members declared in the interface.
Interface can contain methods,properties,indexers and events.
Following interface declares a method called SomeMethod.
interface ISomeInterface
{
void SomeMethod();
}
Someclass implements the ISomeInterface ,so it needs to implement the method declared in the interface.
class Someclass:ISomeInterface
{
public bool void SomeMethod()
{
//implementaion
}
}
Explain what is a constructor in C#?
Constructor is a method of a class which is executed when an instance of a class is created.It is used to perform the class initialization like assigning default values to variables.Though it is just a class method it differs from other methods in these ways
It has the same name as the class name
It does not have any return type
Following is an example of constructor in a class called Employee.Please note that the constructor name is same as the class name,Employee.
class Employee
{
private string _name;
ppublic Employee(string name)
{
_name=name;
}
}
What is method overloading in C#?
Method overloading means having different methods in a class with same name but different signatures.Signature of a method includes its parameter types and name.Its important to realize that return type is not part of method signature.
In the following example there are two method overloads of the sum method.The first method accepts two integer arguments while the second overload accepts three integer arguments.
public int Sum(int a,int b)
{
int sum=a+b;
return sum;
}
public int Sum(int a,int b,int c)
{
int sum=a+b+c;
return sum;
}
Please note that the name of the above two methods is same ,sum.But the two methods differ in the number of arguments making them the overloaded methods.
What is method overriding?
Methods defined in a base class can be overridden in a derived class.This means we can define a method in a derived class with the same signature as the base class.
So we can provide a different implementation of the method defined in the base class.The method which is invoked is determined at runtime depending on the type of object.So if the object reference points to derived class then the derived class method is called and if the reference points to base class then the base class method is called.
class Base
{
public virtual string Hello()
{
return "Hello Base";
}
}
class Derived : Base
{
public override string Hello()
{
return "Hello Derived";
}
}
class Program
{
static void Main(string[] args)
{
Base objBase = new Derived();
string message = objBase.Hello();
Console.WriteLine(message);
objBase = new Base();
message = objBase.Hello();
Console.WriteLine(message);
Console.ReadLine();
}
}
On executing the above code we get the following message
How can we prevent a class from being inherited in C#?
We can prevent class from being inherited by using the Sealed keyword.
sealed class class1 { public class1() { } }
What are the differences between a struct and a class in C#?
Some of the main differences are
Struct is a value type while class is a reference type
Struct variables are stored on a stack while class objects are stored on a heap
We can not inherit a Struct while we can inherit a class
Struct can not have a default constructor(prior to C# 6) while class can have a default constructor
What are the differences between Interface and Abstract class?
- Interface can have only abstract methods while Abstract class can have concrete methods as well.
- All interface members are public
- A class can implement multiple interfaces while it can derive from only single abstract class
What is managed code?
When we write c# code we are writing managed code since it will be executed by the CLR. Manged code gets several benefits like memory management,security.Unmanaged code is the code which is not executed by the CLR.
What is a static constructor in C# ?
Static constructor is used to initialize static data members of a class.It is called as soon as the class is referenced for the first time, whereas an instance constructor is used to initialize an instance of a class.
What is Polymorphism?
Polymorphism is often referred to as the third pillar of object-oriented programming, after encapsulation and inheritance. Meaning of Polymorphism is many-forms .At run time, objects of a derived class may be treated as objects of a base class.This is an example of runtime polymorphism.
What is abstract class?
An abstract class is the one that is not used to create objects. An abstract class is designed to act as a base class (to be inherited by other classes). Abstract class is a design concept in program development and provides a base upon which other classes
What is Dispose?
Its a method invoked for memory cleanup and releasing unmanaged resources like database connections ,file handles.Class’s that wants to perform cleanup operations implements the Dispose method of the IDispose interface.
What is the difference between Dispose and Finalize?
Finalize
Is called automatically by the garbage collector and can not be called by the user code
It is defined by the Object class
There is performance overhead since it is called by the garbage collector at the time of releasing memory used by the object.
Dispose
It is explicitly called by the user code to cleanup unmanaged resources
It is declared in the IDisposable interface
There is no performance overhead
What are constant and read-only variables in C#?
Constants
constant variables must be assigned a value at the time of declaration and it can not be reassigned after this
We can not use static modifier for a constant field
We can declare constant variables inside methods
Readonly
readonly field be assigned a value at the time of declaration or in the constructor after which it can be reassigned
We can use static modifier with a readonly field
We can not declare readonly variables inside methods
What is the use of “using” statement in C#?
The using block is used to obtain a resource and use it and then automatically dispose of when the execution of block completed.
How does CLR execute code?
When the c# code is compiled the output of the compilation is MSIL. MSIL stands for Microsoft intermediate language. MSIL instructions are CPU independent i.e they can run on any CPU.It is the job of the CLR to convert the MSIL instructions into executable code that can be executed on the target machine.
What are the different types of assemblies ?
There are two types of assemblies Private and Shared assembly. A private assembly is used by a single application and is stored in application directory.So it is application specific. A shared assembly is stored in the global assembly cache and can be used by different applications.
What is a NameSpace?
NameSpace Logically group types such as classes and interfaces.The types in the framework are grouped into different namespaces e.g the types in System.Data namespace defines the ADO.NET functionality .We bring all the typed defined in a namespace in scope with using statement.
Can we force garbage collector to run?
System.GC.Collect() forces garbage collector to run.
What is reflection?
All .NET assemblies stores metadata information about the different types defined in the assembly. This metadata information can be accessed by using the classes defined in the “Reflection” API.
What are Value types and Reference types?
Value types directly contain their data on the stack.Reference type variables stores a reference to the data’s memory location and are allocated on the heap.Since value type variable contains the value operation on one one value type variable doesn’t impact the other variable.While reference type variable points to the location of the object so operation on one reference type variable effects all the reference which points to the object.
What is Object Oriented Programming?
Object Oriented Programming is a design concept.In object oriented programming the program consists of objects The objects have a certain relationship with each other and communicate with each other .C# is an object oriented programming language since it supports the object oriented concepts.So a c# program consists of objects which defines state and behavior.
Following are the main object oriented concepts:
- Polymorphism
- Abstraction
- Inheritance
- Encapsulation
Polymorphism
Polymorphism means have multiple forms.So single interface can represent multiple methods.Examples of polymorphism is method overloading and virtual methods.
Abstraction
Abstraction means refining unimportant details and keeping only the required attributes.
Inheritance
Inheritance means inheriting properties and methods from an existing class. Inheritance helps reuse of existing code since a derived class inherits methods and properties from existing class.
Encapsulation
Encapsulation means hiding internal implementation details of a class and providing access to a class through public interface.Encapsulation in C# is implemented using access modifiers.
What is a extension method in C# ?
Sometimes we need to add new functionality to an existing class without modifying the existing class.We may not have the code of the class available or we don’t want to modify the existing class.In such cases we can use extension methods.
Extension method is a method which is added to an existing class without modifying the existing class.To add a new method to a class:
- Declare a static class.
- Declare a static method in the static class.
- The first parameter of the method should use the this keyword.
- The type of the first parameter should be the class type in which you want to add the extension method.

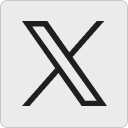

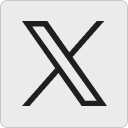

Leave a Reply