Both are used for implementing polymorphism in c#.One is compile time polymorphism-Method overloading.Other is runtime polymorphism-method overriding.
Method Overloading
Here you define two methods in the same class with the same name but different signatures. In the following example Common class defines two method PrintName which are used for printing name of an employee.
First overload takes the name to print while the other overload takes the Employee object as parameter.
class Common { public void PrintName(string FullName) { Console.WriteLine(FullName); } public void Print(Employee emp) { Console.WriteLine(emp.FirstName+emp.LastName); }
Now when you call the PrintName() method then the method to call is determined on the bases of the arguments you pass while calling the method.For example if you use the following method call:
PrintName("Steve")
then first version of the method will be called.
Method Overriding
You define two methods with the same signature,one in derived class and other in base class.In the following example we have declared two classes Manager and Employee.The Employee class defines a Details() method which does not have any parameters.This method just prints the name of the Employee.In the derived Manager class we have overridden this method to print the names of all the employees which a Manager manages.
class Manager: Employee { public List<Employee> Employees{get;set;} public virtual void Details() { foreach(var emp in Employees) { Console.WriteLine(emp.Name); } } } class Manager: Employee { public string Name{get;set;} public virtual void Details() { Console.WriteLine(Name); } }
In method overloading the method to call is determined at runtime,based on the reference used to call the method.If you call the following method then base class method will be called
Employee emp=new Employee(); emp.Details()
If you use the below method call then the derived class method will be called.
Employee mgr=new Manager(); mgr.Details()

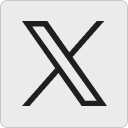
Leave a Reply