C# 6 introduces a new way of handling errors.C# 6 has new keyword when which is used with a catch block.
try catch block is used for error handling in C#.If a error occurs in a try block then control is transferred to the catch block.C# 6 introduces exception filters.Exception filter is applied to a catch block.Exception filter is a predicate which can return true/false values.
- If an exception filter is applied to a catch block the following happens if an error occurs
- If the exception filter evaluates to true value then the corresponding catch block is executed
- If the exception filter evaluates to false value then the corresponding catch block is not executed
The exception filters are quite useful when there are nested method calls.Normally one method calls second method which calls third method and so on.
So assume NestedMethod1 calls NestedMethod2 which calls NestedMethod3. Following is the code for the scenario:
class Program { static void Main(string[] args) { try { MainMethod(); Console.WriteLine("Hello from Main"); } catch(Exception exp) when(true) { Console.WriteLine("Hello from exception"); } } public static void MainMethod() { NestedMethod1(); Console.WriteLine("Hello from MainMethod"); } public static void NestedMethod1() { NestedMethod2(); Console.WriteLine("Hello from NestedMethod1"); } public static void NestedMethod2() { NestedMethod3(); Console.WriteLine("Hello from NestedMethod2"); } public static void NestedMethod3() { throw new Exception(); Console.WriteLine("Hello from NestedMethod3"); } }
Now if an exception occurs inside NestedMethod3 then in a normal scenario the call stack will contain the list of all the methods in the call hierarchy as:
If an error occurs and you are catching the error in main method then the entire call stack is lost:
If you are using when keyword in C# 6 or later then if the when predicate is true only then the catch block is executed.One benefit of this is that you can log the error before handling the exception in catch block:
try { MainMethod(); Console.WriteLine("Hello from Main"); } catch(Exception exp) when(LogError()) { Console.WriteLine("Hello from exception"); }

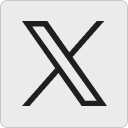
Leave a Reply