System.Linq namespace defines many useful extension methods.Following extension methods are used to return single value from the collection.
- FirstOrDefault
- SingleOrDefault
FirstOrDefault
FirstOrDefault is an extension method defined in the System.Linq namespace.It can be called byt the collections implementing IEnumerable.It returns the default value for the type if the collection doesn’t contain any elements.If the collection contains elements than it returns the first element of the collection.
For example if we declare the collection as:
int[] items = {1,2,3,4,5 };
int returnValue = items.FirstOrDefault();
the returnValue will contain the value 1.Since the collection consists of multilple items it returns the first element
which is 1.
On the other hand if declare the collection as:
int[] items = {};
int returnValue = items.FirstOrDefault();
Since the collection doesn’t contain any items it returns the default value for integer which is 0.
So the returnValue will contain the value 0.
Default value to return for the type is determined by the method default(T).
SingleOrDefault
SingleOrDefault returns a single item from the collection if one element exists for the condition.If more than one element exists for the condition than exception is thrown.
List<int> items = new List<int> { 1,2,3,4,5 };
items.SingleOrDefault(item => item ==1);//returns 1
items.SingleOrDefault(item => item ==0);//returns 0 default value for integer
items.SingleOrDefault();//throws exception
In the above SingleOrDefault method calls, the last call throws exception.Since the items collection consists of more than one elements so the method call returns exception.
First
First returns first matching element from a collection if collection consists of more than one matching elements.
List<int> items = new List<int> { 1,2,3,4,5 };
List<int> itemEmpty = new List<int> {};
items.First(item => item >0);//returns 1 ,first element
itemEmpty.First();//returns exception
The First(item => item >0) method call above returns 1 ,since there are multiple matching elements.The second method call throws exception since the collection doesn’t
contain any elements.
Single
Single returns element if a single matching element is found.If more than one element are found or no element is found than exception is thrown.

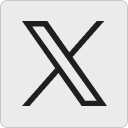
Leave a Reply