There are two ways in which we can execute the LINQ queries ,Immediate and Deferred .Immediate and Deferred query execution in LINQ means when the query is executed.This is because the query declaration in LINQ is different from query execution.
When we write a LINQ query we declare a query variable which contains the result of the LINQ query.
Usually we declare a query variable using var type as:
var results= from student in students where student.class == 12 select student
The above result variable contains the result of the query execution.
You may think that by just declaring the query above ,the result variable will contain the list of students of class
12th.This doesn’t happen.
results variable will not contain the result of query execution until we loop or iterate over the query variable.
class Student { public string Name { get; set; } public string Subject { get; set; } public int Marks { get; set; } public int Class { get; set; } } var students=new List<Student>{ new Student{Name="Amit",Class=12}, new Student{Name="John",Class=10}, new Student{Name="Raj",Class=8}, new Student{Name="Greg",Class=12}, new Student{Name="Priya",Class=12}, }; var results = from student in students where student.Class == 12 select student;
Following iteration executes the query referred by the query variable.
foreach(var student in results) { Console.WriteLine(student); }
This is called deferred query execution
If we want to immediately execute the query we can do so.Immediately query execution will immediately execute the query as soon as we assign the query to the query variable.
We can immediately execute the query by any of the following ways
1.By calling aggregate method such as count.We use this when we need to retrieve a single value such as count of the elements contained in the results collection.
var results = (from student in students where student.Class == 12 select student).Count();
2.By calling the collection methods such as ToList().We use this when we need to convert the results variable to list value such as count of the elements contained in the results collection.
var results = (from student in students where student.Class == 12 select student).ToList();

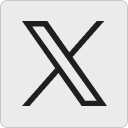
Leave a Reply