Interfaces are important part of C#.Intefaces specifies the services provided by a data type such as class.They specify the method signatures which are defined in the implementing classes.So interface provides details about the methods and other members.
For example if a class implements a specific interface ,then client of that class can use the interface member details to access these.
For example we declare an interface Product as:
interface IProduct { int GetDetails(int id); int UpdateDetails(int id); }
now if any class implements the Product interface then it must implement the GetDetails(int) and UpdateDetails(int) methods.
class ElectornicProduct:IProduct { public int GetDetails(int id) { } public int UpdateDetails(int id) { } }
In this case ElectornicProduct class implements the IProduct interface.This means that ElectornicProduct class implements all the methods of the IProduct interface.
So if a client is aware that a class implements a specific interface then it will be sure that the class implements the members declared by that interface.
If tommorow a new class is added which represents a hardware sold by the company as:
class HardwareProduct:IProduct { public int GetDetails(int id) { } public int UpdateDetails(int id) { } }
We know that HardwareProduct will share common methods with ElectornicProduct as both implements the same interface.Though these classes can add methods of their own but the methods specified in the IProduct interface will be implemented by both these classes.
Important characteristics of interfaces
Following are some of characteristics of interfaces
- Interface does not have any implementation.It is just a specification.There is no method body.The members defined by interface are implicitly public.Though no access modifier is allowed.
interface InterfaceName { ReturnType MethodName(parameters); }
2. A class can implement an interface similarly to how it derives from a base class.Interface name is specified after a colon.A class can implement multiple interfaces.In this case interface names are comma separated.
class ImplementingClass:Interface1 , Interface 2 { public ReturnType MethodName(parameters) { method body } }
A class can derive from a base class and implement interfaces at the same time.The base class name should be last in the list
class ImplementingClass:Interface1 , Interface 2 ,BaseClass { }
3. Following members are allowed in interface
- Methods
- Properties
- Events
- Indexers
We declare property in interface as:
public interface ITestInterface { int Id { get; set; } }
ITestInterface declares an Id property.The get and set accessors do not have any body.The only purpose of declaring property is to indicate the property is readonly ,writeonly or read-write.
Interface can not declare fields or constructors.
4. By convention the names of Interfaces in .NET begins with a capital I.For example the interfaces implemented by collections are called IEnumerable and ICollection.
5. Implementing classes can define the interface members explicitly.Noramly we define interface members as:
class ImplementingClass:Interface { public ReturnType MethodName(parameters) { method body } }
But with explicit interface implementation we implement the interface as:
class ImplementingClass:Interface { public ReturnType Interface.MethodName(parameters) { method body } }
This is useful in the following scenarios:
- If we don’t want to expose the Interface members as public methods of our class.
- When two interfaces declares the members with the same signature
- Our class declares the members with the same name as the interface members
6. Interfaces in c# can be declared as public or internal.By default interfaces are internal.
Programming to Interfaces
There is a concept known as “Programming to Interfaces“.This means that our code refers interface instead of concrete types.
This means that our code will work with any type which implements an interface.For example we can write code which uses an object implementing the IList type.This code will work with all the types which implements the IList interface.
So we declare an array as:
IList collObj=string[5];
collObj variable can be assigned any type which implements IList interface.
If later on we want to replace ArrayList with array we simply need to assign an arraylist to the variable.
IList listObj=new ArrayList();
Our code will work as expected as we have declared the type of collObj variable as IList.

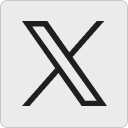
Leave a Reply