In a application logging is a useful feature that allows us to debug the problems in our application.For example an application can write error information to a file in the case of fatal exception.This information can be used for troubleshooting.
Logging frameworks
Logging is the process of persisting run time information.While you are debugging your application ,many times you want to write information at specific points in the code execution.Logging frameworks are useful in such scenarios.Instead of manually writing information you use logging frameworks to manage the different aspects of
the logging such as the logging destination and formatting of the information.
Two main concepts used in logging framework are appenders and formatting the information to save. Appender is used for storing the information to different data sources while formatter is used for formatting the information for storing.Formatting help find the required information easily in the logs.
Though there are different ways by which we can implement logging functionality in our application but it is always better to use a tested solution.log4net is a logging library which is widely used and provides lots of logging features.
log4net provides following features:
- behaviour can be set at run time using a configuration file
- a file, the console, the NT EventLog or even e-mail.
- logging can be enabled or diabled at runtime.
- log4net is thread-safe.
Using log4net in C#
We will see how to implement basic logging functionality using log4net in c#.
Add Nuget package
To install log4net type the following command at the package manager console
Install-Package log4net
If the log4net reference is successfully added then you will get the following result
PM> Install-Package log4net Installing 'log4net 2.0.5'. Successfully installed 'log4net 2.0.5'. Adding 'log4net 2.0.5' to Sample. Successfully added 'log4net 2.0.5' to Sample.
Loggers
Logger object is used to perform the actual logging.It defines methods which can be used to log the different types of information such as information or warning.
Appenders
Appenders are the components which controls the logging such as the destination to which we write the logging information.There are different appenders for different destinations to which we want the information to write.For example ConsoleAppender writes to the console while FileAppender writes to the File.
Logging level
There are five main logging levels which we can use.
- FATAL
- ERROR
- WARN
- INFO
- DEBUG
We define a root configuration section in the config file,which is web.config for web application and app.config for windows application.Appenders are used to append information to some storage location such as file.You can add an appender to root as:
<root> <level value="info" /> <appender-ref ref="FileAppender" /> </root>
Steps to implement logging functionality in c#
Get the logger object
Declare the following log variable at the class level:
private static readonly log4net.ILog log = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
DeclaringTpye passed in the GetLogger method is written to the log.We can give whatever type we want to write to the log
Add the following line to AssemblyInfo.cs
[assembly: log4net.Config.XmlConfigurator(Watch=true)]
The above line let the log4net know that it should look for the configuration information in the main application configuration file.
Add the following in the configuration file
<configuration> <configSections> <section name="log4net" type="log4net.Config.Log4NetConfigurationSectionHandler, log4net"/> </configSections> <log4net> <root> <level value="ALL" /> <appender-ref ref="MyAppender" /> </root> <appender name="MyAppender" type="log4net.Appender.ConsoleAppender"> <layout type="log4net.Layout.PatternLayout"> <conversionPattern value="%date %level %logger - %message%newline" /> </layout> </appender> </log4net>
The line <section name=”log4net” specifies that the log4net configuration information is stored in the config section called log4net which defines the base settings for appenders and the minimum logging level.
The above configuration defines a console appender
We can add a file appender as:
<appender name="FileAppender" type="log4net.Appender.FileAppender,log4net"> <file value="H:\\logfile.txt" /> <appendToFile value="true" /> <lockingModel type="log4net.Appender.FileAppender+MinimalLock" /> <layout type="log4net.Layout.PatternLayout"> <conversionPattern value="%date [%thread] %level %logger - %message%newline" /> </layout> <filter type="log4net.Filter.LevelRangeFilter"> <levelMin value="INFO" /> <levelMax value="FATAL" /> </filter> </appender>
Now we need to get the logger in our code and do the actual logging using the appenders we have defined in the .config file
log4net.Config.BasicConfigurator.Configure(); ILog log = log4net.LogManager.GetLogger(typeof(Program)); log.Debug("This is a debug message"); log.Warn("This is a warn message"); log.Error("This is a error message"); log.Fatal("This is a fatal message"); Console.ReadLine();

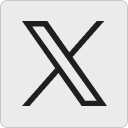
Leave a Reply