Group clause is used in LINQ for grouping collection into groups based on a specific key.LINQ Group By clause in C# returns a sequence of IGrouping<TKey,TElement> objects.
For example if you have a collection of int values then you can group the values based on the integer value.This is a simple example of group by clause.Following list consists of 6 items but there are 4 groups:
List<int> nums = new List<int> { 1, 1, 2, 3, 4, 4 }; var numColl = from num in nums group num by num; foreach(var num in numColl) { Console.WriteLine("num={0} key={1}", num.Key, num); }
If you run the above query then you will get result consisting of 4 groups:
In another example we have declared Employee class which consists of FirstName and joiningDate properties.
class Employee { public string FirstName { get; set; } public DateTime joiningDate { get; set; } }
We are then creating a list of Employee objects:
static List<Employee> employees = new List<Employee> { new Employee {FirstName = "Marc",joiningDate= DateTime.Now.AddHours(1) }, new Employee {FirstName = "John", joiningDate = DateTime.Now.AddHours(1) }, new Employee {FirstName = "Robin",joiningDate= DateTime.Now.AddHours(2) }, new Employee {FirstName = "Mike", joiningDate= DateTime.Now.AddDays(2) } };
Finally we are grouping the employee objects based on the department Employee works:
static void Main(string[] args) { var emps = from employee in employees group employee by employee.Department into empGroup select new { key = empGroup.Key, items = empGroup }; foreach (var item in emps) { Console.WriteLine("group "); Console.WriteLine("Department={0} Total Employeees in department={1}", item.key, item.items.Count()); foreach (var groupItems in item.items) { Console.WriteLine("Employee {0}", groupItems.FirstName); } } Console.ReadLine();
Group items based on multiple keys
In the above examples we have grouped items for single keys only.We can group items based on multiple keys.For example we can group the employee objects based on department and joining date as:
var emps = from employee in employees group employee by new { employee.Department, joinDate=employee.joiningDate > employee.joiningDate.AddYears(-1) } into empGroup select empGroup; foreach (var item in emps) { Console.WriteLine("group consists of following items {0}", item.Count()); foreach (var groupItems in item) { Console.WriteLine("Employee {0}", groupItems.FirstName); } }

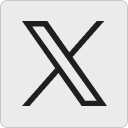
Leave a Reply