LINQ is of different types based on the source of data.For example for querying in-memory data sources or collections LINQ to objects is used.
LINQ to objects is used for querying collections such as List<T>.Any collection which implements IEnumerable<T> or IEnumerable interfaces can be queried using LINQ.
Arrays can be querid using LINQ queries since it implements IEnumerable.
Below is a sample LINQ to Objects query.
string[] users = { "Marc","John","Robin","Prashant" }; var lstUsers = from user in users where user="Marc" select user;
This is called query expression.
We can also write the above query using query operators:
var lstUsers = users.Where(user => user=="Marc") .Select(user);
In the above example we have used the following query operators:
- Where Where operator is used to filter the collection based on the given condition.In this example we have specified the condition that user should be equal to Marc
- Select Select operator is used to project or return values as specified.Here we are returning the user.The return values of the operator is also IEnumerable<T>.
Following are some of the main LINQ query operators:
Use | Query operator |
Filtering | Where |
Projection | Select |
Partitioning | Skip, SkipWhile, Take, TakeWhile |
Ordering | OrderBy, OrderByDescending,ThenBy |
Grouping | GroupBy |
Set | Intersect |

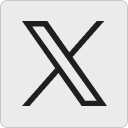
Leave a Reply