Many times you need to initialize class objects while creating them.One obvious approach is that you can use constructors for this.Suppose if you have declared a class as below:
public class Products
{
public int Category { get; set; }
public int Name { get; set; }
}
As you can see it has no constructor,so if you want to create an object of this class and initialize properties at the same time then you can not do so.
But you can initialize object while creating it using the Object initializer syntax.To initialize an objects properties while creating an object you assign values to the properties in curly braces:
var classObject=new Class{Property1=value1,Property2=value2}
Now you can assign values to the properties of the Products class at initialization as:
var product=new Products{Category=1,Name="Desktop"};
we can also initialize collection objects this way >link to another post
Object initializers for creating Anonymous types
Suppose you want to create a class just for using it single time.You can create anonymous class for this.To create anonymous class you use object initializers.For example,if you want to create an object of anonymous class called product:
var product=new {Category=1,Name="Desktop"};
This is specially useful when you are using LINQ and need to project values not defined in a type.In the following example we are projecting values which are not defined in a type:
var items =
from item in items
select new { item.Name,DateTime.Now };

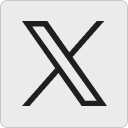
Leave a Reply