LINQ is used for writing queries using C# for querying in memory collections as well as remote data sources.Any data source which implements IEnumerable<T> can be queried using LINQ.It could be any data source which can be queried using LINQ as long as it implements IEnumerable<T> interface.Data source implementing IEnumerable<T> is also called a sequence.
There are different query operators in LINQ which transforms sequence in some way.Some of the query operators are:
- Select
- Where
- OrderBy
select clause is used to project or select the values fromt the given sequence.So in the simplest form you use the range variable in the select clause:
var lst= from rangeVariable in collection where rangeVariable.ID ==1 select rangeVariable;
But many times we want to select only some of the values in the range variable object.We can project only some of the properties in the range variable as:
var lst= from rangeVariable in collection where rangeVariable.ID ==1 select rangeVariable.ID,rangeVariable.Name;
Suppose you want to select custom properties which are not there in the range variable.In such cases you can use anonynous type as:
var lst= from rangeVariable in collection where rangeVariable.ID ==1 select new {ID=rangeVariable.ID+1,rangeVariable.Name+" ."};
In the following example we have Employee object.We want to select only the last two digits of the employee ID since starting five digits are common for all the employees.Also we want to select only the first name from the full name.
Since the required values in the result set are different from the original collection ,we can select only the last two digits and project the values in a new anonymous type.
public class Employee { public string FullName { get; set; } public string Id { get; set; } } var emps = new List<Employee> { new Employee{Id="4000028",FullName="Marc Roberts"} }; //select employees from the list var intermediate = from e in emps select new { Firstname = e.FullName.Split(new char[] { ' ' })[0], ShortCode=e.Id.Substring(4,2) }; foreach(var i in intermediate) { Console.WriteLine(i); }
Instead of the query expression you can project anonymous type using Lambda expression as:
var x= emps.Select(e=> new { Firstname = e.FullName.Split(new char[] { ' ' })[0], ShortCode = e.Id.Substring(4, 2)});

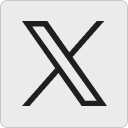
Leave a Reply