List is a generic collection class and can used for storing items of different data types.For using Using Generic List (List<T>)in C# we need to import System.Collections.Generic namespace. List<T> is defined in this namespace.
Creating list class object
To use List<T> object we need to declare and create an object of List type.In the following example we are creating an object of list class of Student type.
List<Student> students = new List<Student>();
Adding elements to list
We can add elements to the student list using the Add method of the list class:
students.Add(new Student { Name = "Amit", Class = 12 }); students.Add(new Student{Name="John",Class=10}); students.Add(new Student{Name="Raj",Class=8}); students.Add( new Student{Name="Greg",Class=12}); students.Add( new Student{Name="Priya",Class=12})
Instead of declaring list object and adding elements in separate lines we can add elements to the list when creating object of List.
var students=new List<Student>{ new Student{Name="Amit",Class=12}, new Student{Name="John",Class=10}, new Student{Name="Raj",Class=8}, new Student{Name="Greg",Class=12}, new Student{Name="Priya",Class=12}, };
We can create List<T> of any type.We can create a List of string type as:
List<string> names = new List<string>();
Iterating list elements using Foreach loop
We can iterate the elements of the list as:
foreach(var student in students) { Console.WriteLine(student); }
An important point to remember is that we can not modify the list elements using the foreach loop.We can just read the value of list elements using foreach loop.
Following are some of the useful methods of the List class:
- Insert(index,item) inserts an item at a specific position in the List
- Remove (item) removes the specified item from the list
- Sort() sorts the items in the list
- Contains(item) checks if an item exists in the list
- ToArray() converts the List to an array
List example
In the following example we are adding elements to the list then using the list methods we are performing different actions:
List<string> names = new List<string>(); names.Add("Amit"); names.Insert(0,"John");//John is the first element in the list names.Sort();//Now Amit is the first element in the list if(names.Contains("Amit")) { Console.WriteLine("name found"); }
Type Safety
One of the advantages that List<T> have is that it provides compile time type checking.For example if we declare a List<string> of string type then we can not add int elements in that List.This prevent run time error which would occur if we don’t use generic list and rather use ordinary arraylist.

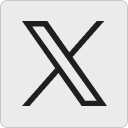
Leave a Reply