Tuples represents data structure which contains a group of values of usually different types.In C# prior to Tuples there was no data type which could be used to represent a group of heterogeneous or different types of values.
A Tuple is a sequence of values.A record in a table is an example of a Tuple.
Tuple has following characteristics:
- Its an ordered sequence of items.
- Every items has a type which is independent of the other items in the Tuple
- Tuple can not be changed once it is created.
For example consider the following method call
static Employee GetEmployeeData(int id) { //return employee data }
If you have to return group of values for the employee then how can you implement it.One possibility is to create a class called Employee which will hold employee data such as:
class Employee{ string Name; string Department; int employeeId; }
Now you can easily populate the employee object in your method and return it to the calling method.
But there is one disadvantage of this approach.You are creating Employee class to just hold multiple values.You will need the Employee class only once.
A better approach to return multiple values from a method in C# is to use Tuple.You can create an object of Tuple class using Tuple constructor or using the Create static method of Tuple class.
Using Tuple.Create method:
var t = Tuple.Create(value1,value2,value3);
Using Tuple constructor
Tuple<datatype1 ,datatype2 ,datatype3>(value1,value2,value3);
As you can see when we use the Create method we don’t need to explicitly specify the data types for different parameters.
You can easily write a method to return multiple values using Tuple as:
static Tuple<string,string,int> GetMultipleData(int id) { if (id == 1) return new Tuple<string, string, int>("Amit", "IT", 1); else throw new Exception(); }
You can call the above method like a normal method:
var employee=GetMultipleData(1);
To access the data returned using Tuple you can use the names Item1,Item2.. for the different fields in Tuple:
employee.Item1 will represent name
employee.Item2 will represent department
employee.Item3 will represent empoyee id

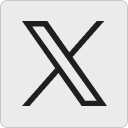
Leave a Reply