Factory Design Pattern
Factory method is a creational pattern used for creating objects.Instead of directly creating class objects by using constructors clients uses the the Factory method to create objects.The client is not aware of the specific class object which it creates by calling the Factory method.
Advantage of Factory Design Pattern
The advantage of Factory Design Pattern is that client is decoupled from the object creation process as it is handled by the factory method.Client just specifies what is its requirement and the object is created and returned to the client by the factory method.So at a high level we can represent this pattern with the following diagram:
Factory Method design pattern is defined as:
Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses.
So according to this definition implementation of this pattern defines an interface which is common to the derived classes.This interface is implemented by the derived classes and each class defines attributes specific to it.
The object creation logic is encapsulated in the factory.The factory creates different objects based on the object creation criteria.
We can understand the Factory Method design pattern using the following diagram.Client uses Creator to create objects.It is important to understand that client uses the Creator ,which is an important component in Factory Method design pattern,to create objects.
Implementation of Factory Method Design Pattern in C#
Now that we have basic understanding of factory design pattern ,we will implement Factory Method design pattern in C#.
We will implement Factory Method Design Pattern in C# by implementing the different components of the Factory Design Pattern as represented in the above diagram.
We define a base class which will represent the different products our factory will create.
abstract class ElectronicProductBase { public string Name{get;set;} public string Memory{get;set;} public string Description{get;set;} }
The ElectronicProductBase is the base class which specifies the common properties of the products which will be created by the factory.Since this class is the base class for Electronic Products so it defines attributes common to all Electronic products such as Memory.
ElectronicProductBase represents the abstract product class in the diagram of factory design pattern above.
Then we define the specific classes based on the ElectronicProductBase base class.MobilePhone ,Laptop and MP3Player are different types of products which will be created by our factory.GenericProduct is the product which will be created if client does not specify any object creation criteria.
abstract class ElectronicProductBase { public string Name{get;set;} public string Memory{get;set;} public string Description{get;set;} } class MobilePhone:ElectronicProductBase { public MobilePhone() { Name = "Mobile Phone"; Description = "Mobile Phone connects people"; } //GSM or CDMA public string PhoneType { get; set; } } class Laptop : ElectronicProductBase { public Laptop() { Name = "Laptop"; Description = "Laptop is a type of computer"; } //DualCore or MultiCore public string ProcessorType { get; set; } } class MP3Player : ElectronicProductBase { public MP3Player() { Name = "MP3 Player"; Description = "MP3 Player is used for listening songs"; } public string SupportedAudioFormats{ get; set; } public string Frequency { get; set; } } class GenericProduct : ElectronicProductBase { }
The different classes represents the different product types which will be created by the factory method.
As these products will be created by the factory so we need to declare the interface of the factory and the concrete implementation.We define the interface as:
interface IProductsFactory { ElectronicProductBase CreateProduct(Products product); }
We declare implementation of the factory interface as:
class ProductsFactory:IProductsFactory { public ProductsFactory() { Console.WriteLine("Creating Products Factory"); } public ElectronicProductBase CreateProduct(Products product) { switch (product) { case Products.Phone: return new MobilePhone(); case Products.Laptop: return new Laptop(); case Products.MP3: return new MP3Player(); default: return new GenericProduct(); } } } //Different products that can be created by the factory enum Products { Phone, Laptop, MP3 }
We have declared Products enum which represents the different products which can be created by the factory.In the following method we loop over the Products enumeration and create different Products by calling the CreateProduct method of the ProductsFactory class.
CreateProduct method returns an object of type ElectronicProductBase.So the client is not aware of the specific Product type since it references the ElectronicProductBase class.This makes the client code decoupled from the actual implementation and does not require any changes if new products are added.
static void Main(string[] args) { ProductsFactory factory=new ProductsFactory(); ElectronicProductBase product; foreach(Products prod in Enum.GetValues(typeof(Products))) { product = factory.CreateProduct(prod); Console.WriteLine("Name: {0} ,Description: {1}", product.Name, product.Description); } Console.ReadLine(); }
So this is simple example of Factory method design pattern in C#.Factory design pattern is one of the creational patterns. Creational patterns such as Factory pattern are useful when you want to handle the object creation process in your application.Factory pattern hides the object creation process from your application.

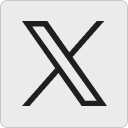
Leave a Reply