- Name Prototype Pattern
- Type Creational Pattern
In this design pattern an existing object is copied to a new object.So we get a new object initialized with the state information from an existing object.The new object that is created using the Original object is different from the original object though it contains the same state information.
Since the objects which are created are based on a prototype,which is the original object, so this pattern is called Prototype pattern.
Following are the uses of the Prototype pattern
- This pattern is useful if we want to create a copy of an object which is expensive or resource intensive to create.Suppose if we are creating an object which contains data which is fetched from a database through a web service.Now we need to create many more identical objects of this type.In this case it is better if we can reuse the existing object rather than creating new objects multiple times.
- We are not required to explicitly set the same properties on a class.Duplication of code is avoided.
Implementation
In the Prototype object define a clone() method.There is an interface called ICloneable which specifies this method.Our prototype can implement this ICloneable interface.
Example of Prototype Pattern
In this example we are taking the example of a School.In a School many students are there and most of the attributes of students are similar.For example students in the same grade will have same subjects.A student has just a few attributes that identifies him.For example student has Name and RollNo which uniquely identifies him.
Since details of Students are fetched from a database so retrieving all the students details from the database could be unnecessary and expensive operation.So we can fetch just the necessary information from the database and use the prototype pattern to create copies of these objects.
We can then fetch the required information for each student such as name and roll number.Since fetching only the required information from the database is less expensive than fetching all the duplicate information so Prototype pattern is a suitable pattern to use here.
abstract class Student { public string Name {get;set;} public string RollNo { get; set; } public string SchoolName { get; set; } public string SchoolAddress { get; set; } public string SchoolStrength { get; set; } public string Principal { get; set; } public abstract Student Clone(); } public class HighSchoolStudent:Student { //Subjects are unique for High School Students public List<Subject> Subjects { get; set; } public override Student Clone() { return this.MemberwiseClone() as Student; } }
HighSchoolStudent is a type of student who belongs to HighSchool.All HighSchool students share the same subjects.The attributes which makes them unique are the roll number and name.
So we will fetch only a single record for a high school student.Then we fetch only the roll numbers and names of all the students.Finally we clone the single high school student that we fetched and assign the name and roll number to the cloned student.
static void Main(string[] args) { StudenService svc=new StudenService(); //GetHighSchoolStudent method will fetch a single record for a high school student Student student = svc.GetHighSchoolStudent(); //GetNamesandRollNo will fetch the names and roll nos of students in high school Dictionary<string, string> nameAndRollNos = svc.GetNamesandRollNo(); foreach(var stud in nameAndRollNos) { Student highSchoolstudent = student.Clone(); highSchoolstudent.RollNo = stud.Key; highSchoolstudent.Name = stud.Value; } }
So this pattern is useful when we want to create many new objects based on some blueprint.This blueprint is specified by the prototype object.

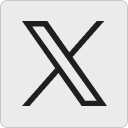
Leave a Reply