Exception handling means that instead of the normal program flow ,program flow goes to exception handling block.Exceptions are raised when an abnormal condition occurs in the program.There could be a condition while the program is executing which doesn’t allow the
program to continue normal execution.Example of such condition is if you try to divide a number by 0.
When an exception occurs then it must be handled in the program.This is provided by the exception handling block.If the excpetion is not handled then program will not be able to run and will terminate.
Raise exception
In the following example an exception will occur and program will terminate.
def convert(): x=0 return 10/x
Console will display the following exception.
ZeroDivisionError: division by zero
The program terminated because we are trying to divide number by 0.Since the exception is not handled program will terminate.
Handle exception
We can prevent the program from being terminated when an exception occur by handling the exception.Python has the try and except constructs for handling exceptions which we can use as:
def convert(): '''x=int(s)''' try: x=0 val=10/x except ZeroDivisionError: return 10/1

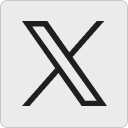
Leave a Reply