Question 1.What is WPF?
Question 2.What are resources in WPF?
Question 3.What are static and dynamic resources?
Question 4.What are value converters?
Question 5.What is MVVM?
Question 6.What is Command in WPF?
Question 7.What is Data binding?
Question 8.What are style triggers?
Question 9.What is PRISM?
Question 10.What are Layout panels?
Question 11.What are Attached properties?
Question 12.What are routed events?
Question 13.What is Dependency Property?
Question 14.What is UpdateSourceTrigger?
Question 15.What is INotifyPropertyChanged?
Question 16.What is XAML?
Question 1.What is WPF?
WPF stands for Windows Presentation Foundation.It is the successor to windows forms for developing windows applications.It provides several advantages over windows programming which has been used previously for developing windows applications.
It has the following advantages:
- Use of Hardware acceleration.Windows Forms uses GDI which is an old technology for performing Graphic related activities.WPF uses DirectX in which the responsibility of rendering of the application is shifted from CPU to GPU.
- Uses resources and styling to reuse the resources and define consistent look and feel across the entire application.
- Hardware and Resolution independent
- WPF uses XAML to define the UI.In Windows Forms applications whenever we change UI ,in background Designer.extension code file is updated.In contrast WPF uses just XAML to design the UI.As XAML is a declarative language,so code and UI are decoupled.
- WPF provides features which helps build application using MVVM pattern.
Question 2.What are resources in WPF?
Resource is used to reuse the data in a WPF application.We define resources in our application ,either globally or locally once and then reuse it from multiple places in the application.So instead of defining data and properties multiple times for different controls and views we can define it once as a resource.This helps in maintenance and re-usability.
Styles and templates are commonly defined as resources.
Following is an example of a resource:
<Page.Resources> <Style TargetType="TextBlock" x:Key="CommonTextStyle"> <Setter Property="Background" Value="Blue"/> </Style> <TextBlock Style="{StaticResource CommonTextStyle }">Title</TextBlock>
Question 3.What are static and dynamic resources?
The main difference is that static resources are evaluated at compile time while dynamic resources are evaluated at run time.
Static resources uses StaticResource Markup Extension while dynamic resources uses the DynamicResource Markup Extension.If you need to update the resources at run time then you should use dynamic resources.
Question 4.What are value converters?
Sometimes we need to convert the data from one type to another type.This could be required when you are binding two properties which have different data types.Vaue converters are used in such a scenario to convert the value from one data type to another.
Value converter is a class which implements the IValueConverter.
public class SampleConverter : IValueConverter { public object Convert(object value, Type targetType,object parameter, CultureInfo culture) { // Do the conversion } public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture) { // Do the conversion from visibility to bool } }
Question 5.What is MVVM?
MVVM is a architectural pattern which divides the application into three layers:
- View Responsible for defining the UI of the application
- Model Business logic and domain entities
- ViewModel Connects the view with the model
The advantage of MVVM pattern is separation of concerns.Since each layer is loosely coupled to the other layers we can easily change any of the layers without affecting the rest of the application.
Question 6.What is a Command in WPF?
Command encapsulates the request for an action.This command object can be passed to the component which will handle the request.This decouples the request for an action from the request handler.
Command can be used in XAML to bind event to the event handler logic in ViewModel using markup extension.
Question 7.What is Data binding?
UI elements such as textbox are bound to the ViewModel properties through data binding.There are three types of bindings:
Question 8.What are style triggers?
We can set the properties of the UI elements when certain condition is true.For example we can set the background color of textbox when it is focussed.We can use trigger to change the properties of the elements dynamically.
For example below trigger will set the background color of the WPF textbox to gray when it is focussed.
<Style.Triggers> <Trigger Property="IsFocused" Value="True"> <Setter Property="Background" Value="Gray"/> </Trigger> </Style.Triggers>
Question 9.What is PRISM?
PRISM also called composite application block is used for developing application which consists of different components.The components are connected together to create the application.Different components can be developed separately by different teams.Since components are loosely connected using dependency injection so different modules or components can be worked on independently by different developers.
Question 10.What are Layout panels?
Layout panels are used for arranging other controls.They handle the positioning of the controls.
Layout panels
- Grid Panel consists of rows and columns.
- Stack Panel positioning controls horizontally or vertically
- Dock Panel positioning controls top,bottom,horizontally or vertically
- Wrap Panel positioning controls sequentially
- Canvas Panel positioning controls based on fixed coordinates
Question 11.What are Attached properties?
Attached properties are kind of dependency properties.They differ from the normal dependency properties.Attached properties are not used in the same class where they are defined.The class which defines the dependency properties allows its children objects to set the value of attached properties.For example layout panels such as grid panel and stackpanel defines different properties.These properties are available to the children objects even though they are defined by these panels.
Question 12.What is Dependency property?
WPF dependency properties are a wrapper around normal CLR properties.They provides extra features to the normal CLR properties.Dependency properties provides features such as:
Value of dependency property depends on several sources such as animation,styles and user input.Each source has a different priority when determining the value of dependency property.
The value of a normal CLR property is read form a private variable.The value of dependency property is read using the GetValue() method of the DependencyObject class.A class which defines dependency property should inherit from DependencyObject .
Question 13.What is Routed event?
Routed event allows us to handle the event at the different source where the even originated.The routed event is passed to the tree of controls until it is handled.Routed event are of the following types:
Tunnelling events These first raised for the root element such as the outermost window,then it is raised for the other controls in hierarchy and finally raised for the control where the even originated
Bubbling events These are opposite of Tunnelling events.These are raised first for the control where the event originated and then they are raised for the other controls.
Direct events This is the same behaviour as a normal event.The event is raised only for the originating control
Question 14. What is UpdateSourceTrigger?
In WPF two way data binding, the changes in the target property are propagated to the source property.When the changes should propagate depends upon the UpdateSourceTrigger property.This defines the event in the target object that should update the bound property in the source object.For example if the UpdateSourceTrigger of a TextBox is lostfocus then the source property will be updated when the TextBox loses focus.
What is INotifyPropertyChanged interface?
When UI is bound to the data objects ,then when the property value in the data object changes ,the UI needs to be notified.INotifyPropertyChanged interface is used to notify client or the UI about the changed property value.It is defined in the System.ComponentModel namespace.It defines a single event PropertyChanged.When the property value changes you need to raise the PropertyChanged event.
For example if you have a Total property then you will raise the PropertyChanged event as:
public int Total { set{ _total=value; PropertyChanged(this, new PropertyChangedEventArgs("Total")); } }
XAML(pronounced as Zammel)stands for Extensible Application Markup Language.It is a markup language based on XML.It is developed by Microsoft.XAML is used for developing UI of WPF application.

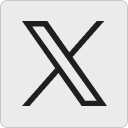
Leave a Reply