The browser toolbar is one of the most important UI element in any web application.One of the most common ways to navigate between the different pages is through the back and forward buttons on the toolbar.
What the back and forward buttons actually do is that they help to navigate between the pages already visited by us.The pages which we have visited are stored in the browser history.So clicking the back button instructs the browser to go back in the list of history entries while clicking the forward button instructs the browser to go forward in the history.
In the previous versions versions of HTML we can easily navigate between the browser history using the back(), forward(), and go() methods.
To move backward through history we can use the back() method as:
window.history.back();
The above method works as if the user clicked the Back button on their browser toolbar.
To move forward we can use the forward() method as:
window.history.forward();
Though we can easily navigate the browser history using the above methods , in the previous versions of HTML there was no easy way to manipulate the browser history.One questions you may ask is what is the need for manipulating the browser history ?.To answer this question just think about how we make requests to the web server in our applications.
- Frequently we directly type the URL in the browser address bar
- The different pages in our applications can also request a resource at a different URL by providing hyperlinks which the user can click for navigating to the specific page
- We partially update a page using the AJAX
Suppose we do a partial page update using AJAX and update our page.Now if we click the back button we may not navigate to the previous state of the page but rather to the previous URL which can even be a URL outside our application.
It is the last point ,making ajax requests,which can benefit most by using the HTML5 History API .We often have faced this problem in the past when we had implemented some ajax functionality in our application and after updating the page contents the back and forward buttons no longer works as expected.
If we implement the History API in a application which uses AJAX for updating the page contents then from the end users perspective the experience will be similar to visiting a new URL.
Following will happen now
- User clicks on a button which requires to fetch some content from the server
- Browser makes an ajax request to the server for fetching the content
- Server responds with the content,browser updates the page with the new content from the server
Once the above steps completes if the user clicks the forward and back buttons the page will get updated with the correct content,If the user clicks the back button he will be able to view the previous content while if the user clicks the forward button the page will be updated with the updated new content.Also the browser URL will be updated as we click the back and forward buttons.
Let’s see how we can achieve the above functionality using the HTML5 History API.
To manipulate the history entries in the browser two new methods are provided by the History API
- pushState Adds a new history entry
- replaceState Replaces an existing history entry
Both the pushState and replaceState methods have the same arguments but they differ in their usage.
Apart from the above methods there is an event onpopstate which is useful for working with the browser history.
We use the pushState method as:
history.pushState(state,title,url)
state : object represents the state of the page.This object is passed to the onpopstate event.We can use this object to change the state of the page depending on the history entry selected.It is important to note that we need to explicitly set the state of the page on selection of history entry ,for which we can use this state object.
We pass a javascript object in this parameter.We can define the values in this object which we want to retrieve when the user navigates back to this history entry.Suppose page is displaying data for an employee named John having employee id “1” so we can save this information in the state parameter as:
var state = { name: "John" }; history.pushState(state, "title", "employees/1");
We have provided relative URL above.So if the full URL of our application is www.organization.com so our new URL will be www.organization.com/employees/1
title :represents the title of the page.As currently browsers don’t support this so we can ignore it as of now.
url : the URL for the history entry.This can be relative url to the current url or it can be absolute url.If we don’t specify URL then this is set equal to the current url.
Calling the pushState method will add an item in the list of history entries and we can navigate through the history entries using the back and forward buttons.But though clicking the back and forward buttons will navigate to the corresponding entry in the list of history entries but state of the page will not be automatically updated.To update the state of the page for the history entry we use the popstate event.
The popstate event is called whenever the user navigates to a history item.We can attach the popstate event to the window as:
window.addEventListener('popstate', function(event) { // event.state contains the state information });
replaceState method is similar to the pushState method and expects the same parameters but instead of making a new entry in the list of history items it updates the current history entry.

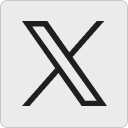
Leave a Reply