Most of the applications today works with files such as word documents or pdfs.So one of the common requirements in most applications is uploading files.In the case of windows applications uploading files could be very fast as the user is working with files stored locally on his machine.
Uploading files in web applications could be a time consuming process since the file is on the local machine of the user and it is required to be sent with the HTTP request to the server.Since this process could take significant time ,implementing file upload functionality using ajax improves the user experience while file is being uploaded on the server.
We need to follow the below steps when implementing file upload functionality using ajax
- Declare a file input type
<input type="file" id="fileinput" />
2. Create an instance of FormData
FormData is used for passing key value pairs to the server in an HTTP Request.We create an object of FormData and add the file in it.
var formData = new FormData(); formData.append('file', file);
3. Pass the formdata object in the request
We pass this formdata object to the server using $.ajax() method of jQuery
function uploadFile(file) { var formData = new FormData(); formData .append('file', file); $.ajax({ url: "InsertFile/", type:"POST", data: formData , cache: false, contentType: false, enctype: 'multipart/form-data', processData: false, success: function (data) { }, error: function (xhr, status, error) { } }); }
4. Read the file in the action method
Finally we read the posted file in the action method.The uploaded file will be available as a parameter of type HttpPostedFileBase.We can read the file using this object.We can perform different operations using this object such as saving file in the database.
[HttpPost] public ActionResult InsertFile(HttpPostedFileBase file) { BinaryReader binaryReader = new BinaryReader(file.InputStream); byte[] binaryData = binaryReader.ReadBytes((int)file.InputStream.Length); string result = System.Text.Encoding.UTF8.GetString(binaryData); // Logic to insert the file in database return View(); }

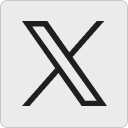
Leave a Reply