Web applications of today are becoming as much responsive and user friendly as the desktop applications as more and more processing is being done on the client side.Performing tasks on the client means more computations need to be performed on the client’s machine.
With the introduction of javascript frameworks like jquery and angularJS we can easily create a complex application in which much of the application logic resides on the client side.
But doing more processing on the client’s machine has one big consequence.If the computation is complex enough to consume more processing time,the end user may be blocked to perform any other operation.It is not uncommon to see dialog box such as below due to unresponsive web page.
Non-responsive web page dialog in Chrome
The reason for the unresponsive web page is that javascript runs in a single thread,which means that as one javascript command is executing the next command will have to wait ,as the code executes sequentially.So a computationally complex operation may block the rest of the code from executing.
Web workers provides a means of executing javascript code in separate threads.So a web worker is a means of bringing multithreading support to javascript programming.
Because of this browser can execute multiple tasks at the same time.As the processing power of the client’s machines is increasing day by day ,so it is not uncommon to have a multicore processor which can really use the multithreaded programming model provided by webworker.
Workers can be of two types dedicated and shared.The difference is that a dedicated worker can be accessed only by the code which has created it while shared worker can be accessed by any script.Here we will be considering only the dedicated worker.
Implementing worker which executes javascript in our web page is a simple.We just need to follow the below steps:
1.Check if the browser supports worker.To check if the browser supports dedicated worker we can use the following code.
if (window.Worker) { /*browser supports worker*/ }
2. Create a .js file which contains the javascript code that we want the worker to execute.
var count = 0; function incrementCount() { count = count + 1; postMessage(count); /*pass the response to web page */ }
3.Create worker object and the pass the name of the file created in step 1 above as an argument to it’s constructor.
var worker = new Worker('worker.js');
4. We start the worker by calling the postMessage() function on the worker object.
worker.postMessage();
The above steps are sufficient to create a webworker and start it.There are few other things which we can do while working with the webworker object.
- We may need to pass data from the web page to the webworker.
- We may need to receive data from the webworker in the calling webpage.
- We may need to terminate the worker object once we are done using the object.
5. Passing the data from the WebPage to the worker
To pass and receive data we use event handling and the postMessage() function.We pass the data to the worker as an argument to the postMessage() function.
worker.postMessage("Hello worker!")
6. Receiving the data from the worker in the WebPage
To receive the data passed from the worker in the webpage we use the onmessage event.evt.data contains the data passed by the worker.
worker.onmessage = function OnMessageReceived(evt){ alert("Message from worker: " + evt.data); }
7. Terminating the webworker
Once we are done using the worker ,its important to terminate the worker object otherwise it will continue to run and consume the system resources.To terminate the worker object we just need to call the terminate method on the worker object
worker.terminate();
Web Workers in HTML5 brings multithreading programming model to the client side.This helps in creating asynchronous applications.Tasks which require heavy processing can be done in a separate thread while the main thread can process UI events.

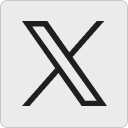
Leave a Reply