In this example we will see how to connect push button to raspberry pi.The push button will act as an input interface to raspberry pi.
To implement the circuit fort the application we will need the following:
- breadboard
- jumper wires (2 male to female)
- push button
- raspberry pi (b+ in this example)
set up the circuit as:
attach the push button on the breadboard such that two terminals are together and 2 are separated.You need to use different unconnected rows in the breadboard.
connect the jumper wires
- GPIO Grd(PIN 3) to one terminal of push button
- GPIO 16 to other terminal of push button
Following is the layout of the raspberry pi GPIO (3 B+).
Following is the Python code:
import RPi.GPIO as GPIO import time GPIO.setmode(GPIO.BCM) GPIO.setup(16, GPIO.IN) if GPIO.input(16) == False: # you can also use 1/0 instead of true/false print('I am pressing the button') time.sleep(0.2)
There is one important point to note here,the call to setup() method:
GPIO.setup(16, GPIO.IN)
What this does is set the GPIO pin which you want to use.Here we are using the GPIO pin 16 ,but you can use another GPIO.
and we are reading the input using the GPIO.input() method.This method return boolean values ,TRUE/FALSE or 1/0.
Inputs are Boolean values: 1 or 0, GPIO.HIGH or GPIO.LOW, True or False.
You can save the above script in a file called pushButon.py and execute it as:
python pushButon.py
If you run the above code and press the button then the following text will be printed:
I am pressing the button

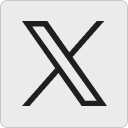
Leave a Reply