Here we will implement a simple circuit using Raspberry PI GPIO for blinking LED after every few seconds.You will need the following to implement this:
- Breadboard
- Raspberry PI
- Resistor
- Led
- Two male to female jumper wires
First you will need to make the connection the breadboard.
In breadboard make the following circuit connection:
- attach the led to breadborad:The long pin of led is anode(+ev) and short leg is cathode(-ev).
- attach one end of resistor in same column as the anode(long leg) of led and attach the another end to another point in same row.
- attach jumper wires:attach one jumper wire from short leg of led to ground GPIO(one of the GPIO: 6,14,20,39,25) in raspberry pi. attach another jumper wire from another end of resistor to GPIO 5( you can use any other GPIO)
To implement Windows 10 IoT Core app you need to create UWP app.
After you create a new UWP app you need to modify the MainPage.Xaml as:
Import the following namespace in the MainPage.xaml page:
using Windows.Devices.Gpio;
declare variables of type GpioPin and GpioPinValue as:
private GpioPin gpioPin; private GpioPinValue gpioPinValue;
Now we need to initialize the GPIO pin we have declared above.We can initialise it in the Init() method as:
private void InitGPIO() { var gpioController= GpioController.GetDefault(); gpioPin = gpioController.OpenPin(5); gpioPin.Write(GpioPinValue.High); gpioPin.SetDriveMode(GpioPinDriveMode.Output); }
We are doing following here:
- retrieving gpioController object using the static GetDefault() method of the GpioController class
- Updating the pin with a value using the Write() method.We are setting the value GpioPinValue.High value of the GpioPinValue enum
- Finally we are setting the mode of the pin as output
private void time_elapsed(object sender, object e) { if (gpioPinValue == GpioPinValue.High) { gpioPinValue = GpioPinValue.Low; gpioPin.Write(gpioPinValue); LED.Fill = redBrush; } else { gpioPinValue = GpioPinValue.High; gpioPin.Write(gpioPinValue); LED.Fill = grayBrush; } }
Now we need to define a method which will be called every 5 seconds.This method will be called after every 5 seconds and it will reverse the current value of GPIO pin.
private void time_elapsed(object sender, object e) { if (gpioPinValue == GpioPinValue.High) { gpioPinValue = GpioPinValue.Low; gpioPin.Write(gpioPinValue); LED.Fill = ledMainBrush; } else { gpioPinValue = GpioPinValue.High; gpioPin.Write(gpioPinValue); LED.Fill = ledDimBrush; } } private SolidColorBrush ledMainBrush = new SolidColorBrush(Windows.UI.Colors.Blue); private SolidColorBrush ledDimBrush = new SolidColorBrush(Windows.UI.Colors.LightGray);
Following is the complete sample:
public sealed partial class MainPage : Page { private GpioPin gpioPin; private GpioPinValue gpioPinValue; private DispatcherTimer timer; private SolidColorBrush ledMainBrush = new SolidColorBrush(Windows.UI.Colors.Blue); private SolidColorBrush ledDimBrush = new SolidColorBrush(Windows.UI.Colors.LightGray); public MainPage() { InitializeComponent(); timer = new DispatcherTimer(); timer.Interval = TimeSpan.FromMilliseconds(200); timer.Tick += time_elapsed; InitGPIO(); if (gpioPin != null) { timer.Start(); } } private void InitGPIO() { var gpioController = GpioController.GetDefault(); gpioPin = gpioController.OpenPin(LED_PIN); gpioPinValue = GpioPinValue.High; gpioPin.Write(gpioPinValue); gpioPin.SetDriveMode(GpioPinDriveMode.Output); GpioStatus.Text = "GPIO pin initialized correctly."; } private void time_elapsed(object sender, object e) { if (gpioPinValue == GpioPinValue.High) { gpioPinValue = GpioPinValue.Low; gpioPin.Write(gpioPinValue); LED.Fill = ledMainBrush; } else { gpioPinValue = GpioPinValue.High; gpioPin.Write(gpioPinValue); LED.Fill = ledDimBrush; } }

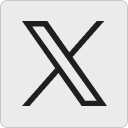
Leave a Reply