jQuery has many different types of widgets which makes it easy to add different types of functionalists in our applications.One commonly used widget is Datepicker. Most of the applications provides user the option to select the dates.So instead of allowing the user to select date in any arbitrary format which is also inconvenient for the user, we can use the datepicker widget which makes it easier to select the date from a calendar.
We can easily add and customize the Datepicker widget.To add the datepicker in our application we need to add the required jQuery libraries and the css.We need the following jQuery libraries to use the datepicker widget
- jQuery core library
- jQuery ui library
- jQuery ui css
We can easily add these using the googleapis.Below we have included the required libraries and css
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.6/jquery.min.js" type="text/javascript"></script> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js" type="text/javascript"></script> <link href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/themes/base/jquery-ui.css" rel="Stylesheet" type="text/css" />
Once we have added the above libraries we add the input element we want to associate the datepicker with .Finally we call the datepicker() on the input element. Below we are adding the widget to a birthdate input field.
We add the input field with id birthDate
Date<input type="text" id="birthDate" />
We can now associate the datepicker widget with the field by using the below jquery snippet.
$(function () { $("#birthDate").datepicker(); } )
If we now request our html page we will get a textbox on the page.On clicking or focusing on the text field a calendar is displayed from which the user can select the date.
Once the user select the date from the calendar ,the calendar popup is dismissed and the selected date is populated in the textbox.
Above we have added the datepicker widget with just the default functionality.Datepicker gives us lot of options for customization.
Some of the options for customizing the datepicker are
showOn This option is used to specify how we want to trigger popup calendar.The values we can specify for this can be
- focus Displays the calendar when the input field receives focus.
- button Displays the calendar when the button is clicked.This option also adds a button adjacent to the input control.
- both Displays the calendar on both the click of the button and when the input field receives the focus.
In the following code we have specified the value of both so that the calendar will be displayed both on click of the button and when the input field receives focus.Also we have set the text for the button using the buttonText option.
$(function () { $("#date").datepicker({ numberOfMonths : 3, showOn: "both", buttonText:"select", appendText: "(yyyy-mm-dd)" }); } )
numberOfMonths This option is used to specify how many months are displayed when the calendar is displayed .By default only a single month is displayed ,by specifying this value we can control how many months are displayed at a time.
changeMonth and changeYear By default the datepicker displays a calendar with previous and next arrows only.If we want to select years and months which are in the distant past or future using previous and next arrows is not very easy to select such years and months.We can set changeMonth and changeYear options to true to display dropdowns for years and months.By using the drop downs for years and months user can easily select any year and month.Below we have set the changeMonth and changeYear as true.
$("#date").datepicker({ changeYear: true, changeMonth: true, });
On executing the above script following calendar is displayed by the datepicker when user focuses on the input field.
dateFormat We can display the dates in the input field when the user selects the date from the calendar in different formats using the dateFormat option.Different characters represent different date format options. Some of the date format options are
[table id=4 /]
If we want to display the selected date in the format 24-December-14 we can use the below date format
$(function () { $("#date").datepicker({ dateFormat : 'd-MM-y' }); } )
Displaying as Inline
Instead of displaying the datepicker on clicking or focusing on an input field we can embed the datepicker in the page so that it is visible all the time.To create an inline datepicker we need to call the datepicker() on the div instead of an input field.
$(function() { $( "#reservationDate" ).datepicker(); }); Reservation Date: <div id="reservationDate"></div>

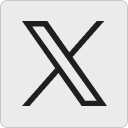
Leave a Reply