In javascript we use the alert or confirm message box to display message to the end user.Though it is the commonly used way to show informational message to the end user it has the following shortcomings
- Message box may defer from browser to browser
- It is not easy to customize the fixed layout of the message box
Below the javascript alert box is displayed in chrome and Internet explorer.As you can see their layout is not consistent.
Javascript alert in Chrome
Javascript alert in IE
Also we can not change the layout of the inbuilt dialog boxes.An alert box will always contain a button with “OK” text and a message ,we can not add an extra button in an alert box if we require.The jquery dialog provides a convenient way to display a consistent message box and and we can control its layout .
To add the jquery dialog to the page we need to follow the below steps
Add the jQuery library and jQueryUI plugin.We can use the google cdn to add these
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.6/jquery.min.js" type="text/javascript"></script> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js" type="text/javascript"></script> <link href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/themes/ui-lightness/jquery-ui.css" rel="Stylesheet" type="text/css" />
Add a div which contains the content we want to display in the dialog
<div id="dialogBox" title="Dialog Box!"> Hello! </div>
Attach the dialog to the source div
Once we have defined the div we can call the dialog() widget on the div.Normally we need to call the dialog() in some event handler.
$('#dialogBox').dialog({ }) ;
We will get the below dialog if we follow the above steps
We have created a dialog which just displays a message to the user.Dialog in jQuery provides many customization options.Some of the options available are
buttons To add buttons to the dialog.The buttons property takes a javascript object as its value.The javascipt object contains the button text and the function that we want to associate with a button.We can add a button to the dialog box as
buttons: { "Confirm": function () { //confirm button logic }
modal To specify that the dialog is modal.Modal dialog means that the user must select any option in the dialog.The modal dialog get the focus and the background is disabled until user selects some value from the dialog.
The background is disabled in Modal dialog
title To specify the title of the dialog.If we don’t specify this value then the title value present in the source ,such as div ,will be used.Though we have not spcified any value for the above dialog even then the title is displayed in the above dialog as the title is present in the source div.
<div id="dialogBox" title="Dialog Box!"> Hello! </div>
If not specified by the dialog widget the title of the source is used
closeOnEscape This specifies that if the dialog has the focus and escape key is pressed then the dialog closes.
show Specifies how to animate the opening of the dialog.If set to false then no animation will be used.If we specify the number then the dialog will display within the specified duration in milliseconds.Default value is null.
hide Specifies how to animate the close of the dialog.If set to false then no animation will be used.If we specify the number then the dialog will close within the specified duration in milliseconds.Default value is null.
jquery dialog with hide value set to 1000.
Close This method closes the dialog.If we need to close the dialog using the script then we can call the close() method.
isOpen This method returns a boolean value which indicates whether the dialog associated with the element passed as an argument is currently open.To check if the dialog associated with a div with id “message” is open we can use
$("#message").dialog( "isOpen" );

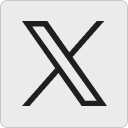
Leave a Reply