A common requirement when working with arrays is to loop over the array elements. $.each() is a useful method for iterating over the array elements.We can also specify the action to perform for each element using a function.We pass this function as an argument to the $.each().
To process all the elements in an array we use the $.each() as:
$.each(arrayName,function(index,value){ });
first parameter index represents the index of the array element ,second parameter value represents the value of the array element.
For example we have declared an array of countries.The countries are added to the array in a sequence of their rank in a sports event.
var cars = ["Singapore", "UK", "US","Australia","Germany","France"];
For working with list of elements as above $.each() is quite useful.
To display the array above in a table we can use $.each() as:
$(document).ready( function () { var countries= ["Singapore", "UK", "US","Australia","Germany","France"]; $.each(countries, function (index, value) { if (index == 0) { $("#countriesTable").append("<tr style='background-color:blue'><td>" + index + "</td><td>" + value + "</td></tr>"); } else { $("#countriesTable").append("<tr><td>" + index + "</td><td>" + value + "</td></tr>"); } } ) ; });
In the above script we are appending the countries as rows in a table.If the index of the country is 0 that means it is ranked first so we display it in blue color.
We declare the table to which we add countries rows as:
<body> <table width="300" border="1" id="countriesTable"> <tr> <th>Rank</th> <th>Country</th> </tr> </table> <div></div> </body>
On executing the above script we get the following list of countries
There is one more type of each() function.Apart from calling the each() function using the $ identifier ,each() function can also be called using the $() function.
Its syntax is:
$(Element Selector).each(function(index,element))
When used like this each() function will be called for every matching element.

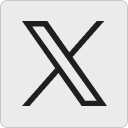
Leave a Reply