JSON is an object which contains methods for converting between JSON and their string representation.We can not create an object of JSON ,instead we call the methods directly.The two main methods it contains are parse and stringify.
It is important to validate if a browser supports the JSON object as it might not be supported by very old browsers.Modern browsers such as IE 8+,Chrome 3+ supports this object.
Convert string to Javascript Object
JSON defines parse method for converting from string to object.For example a object can be represented in string format as:
var person='{ "Name":"Marc","Age":"20"}'
The above string represents the object using the JSON syntax as name value pairs.It can be converted into actual object as:
var personObject=JSON.parse(person);
at runtime the value of personObject will be as:
Object {Name: "Marc", Age: "20"}
If the string which we pass to the parse method doesn’t represent a javascript object then exception will be thrown.So it is important to pass a valid string to this method.
Convert Javascript object to string
For converting from Javascript object to string there is a stringify method which expects an object.To convert the personObject we created above ,stringify function can be used as:
var personObject = JSON.stringify(personObject);
At runtime the value of personObject will be:
"{"Name":"Marc","Age":"20"}"
It represents the object using the JSON syntax of keys and values.
Replacer function
Both parse and stringify methods takes an optional argument ,a replacer function.This function is invoked whenever an object is serialized or deserialized.
We use it as:
var object = JSON.parse(json, function(key, value) { if ( key == 'TestKey') return 'Sample value'; } );
JSON parse and stringify methods are useful if the browser supports the JSON object.But in browsers which doesn’t supports this object we can use the parseJSON(string) method of jQuery for converting string to object.
var jsonObject = $.parseJSON(string)

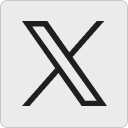
Leave a Reply